解释a = (1-((i*torch.pi)**2)) ** 0.5
时间: 2024-08-14 09:06:55 浏览: 80
这段代码是在PyTorch库中使用的,它涉及到张量操作和数学表达式。这里的`a`是一个变量名,`i`可能是某个索引或者循环变量,`torch.pi`代表π常数(类似于numpy中的π),`**`表示幂运算。
首先计算`(i * torch.pi) ** 2`,即`i`乘以π的平方,然后从1中减去这个结果,得到`1 - ((i * torch.pi) ** 2)`。接着,对这个差值求平方根,所以整个表达式`(1 - ((i * torch.pi) ** 2)) ** 0.5`的结果是一个开方后的数值,可能用于模型训练中的某些数学变换或正则化。
简单来说,这段代码是对每个`i`对应的`((i * π)² - 1)`取平方根。如果你想了解更多关于这在具体场景下的应用,例如神经网络权重衰减或者其他优化算法,可能需要更多的上下文信息。
相关问题
pytorch PINN求解分段初边值条件不为sin(pi*x)的Burger方程的间断问题的预测解和真实解以及误差等值线图的代码
以下是求解分段初边值条件不为sin(pi*x)的Burger方程的间断问题的预测解和真实解以及误差等值线图的代码:
```python
import torch
import numpy as np
import matplotlib.pyplot as plt
# 设置随机数种子,以便结果可重现
torch.manual_seed(1234)
np.random.seed(1234)
# 定义Burger方程
def f(u, v, x):
u_x = torch.autograd.grad(u, x, grad_outputs=torch.ones_like(u), create_graph=True)[0]
v_x = torch.autograd.grad(v, x, grad_outputs=torch.ones_like(v), create_graph=True)[0]
return u*v_x + 0.01*torch.autograd.grad(v_x, x, grad_outputs=torch.ones_like(v_x), create_graph=True)[0] - 0.1*u_x
# 定义边界条件
def g_left(t):
return 0.5*(torch.tanh(20*(0.25-t)) + 1)
def g_right(t):
return 0.5*(torch.tanh(20*(0.75-t)) + 1)
def h(u):
return u
# 定义PINN模型
class PINN(torch.nn.Module):
def __init__(self):
super(PINN, self).__init__()
self.fc1 = torch.nn.Linear(1, 50)
self.fc2 = torch.nn.Linear(50, 50)
self.fc3 = torch.nn.Linear(50, 50)
self.fc4 = torch.nn.Linear(50, 1)
def forward(self, x):
h = x
h = torch.nn.functional.relu(self.fc1(h))
h = torch.nn.functional.relu(self.fc2(h))
h = torch.nn.functional.relu(self.fc3(h))
h = self.fc4(h)
return h
# 定义训练函数
def train(model, x_left, x_right, x_inner, y_left, y_right, optimizer, epochs):
losses = []
for epoch in range(epochs):
optimizer.zero_grad()
# 计算边界损失
y_pred_left = model(x_left)
y_pred_right = model(x_right)
loss_boundary = torch.mean((g_left(0) - y_pred_left)**2) + torch.mean((g_right(0) - y_pred_right)**2)
# 计算内部损失
u = x_inner[:, 0]
t = x_inner[:, 1]
u.requires_grad = True
t.requires_grad = True
v = model(u)
f_pred = f(u, v, t)
loss_interior = torch.mean((f_pred)**2)
# 计算总损失
loss = loss_boundary + loss_interior
# 反向传播和优化
loss.backward()
optimizer.step()
# 记录损失
losses.append(loss.item())
# 打印中间结果
if epoch % 1000 == 0:
print("Epoch {}: Loss {}".format(epoch, loss.item()))
return losses
# 生成训练数据
n_left = 50
n_right = 50
n_inner = 2000
x_left = torch.linspace(0, 0.25, n_left)[:, None]
x_right = torch.linspace(0.75, 1, n_right)[:, None]
x_inner = torch.rand(n_inner, 2)
x_inner[:, 0] = x_inner[:, 0]*0.5 + 0.25
x_inner[:, 1] = x_inner[:, 1]*2
y_left = g_left(0)
y_right = g_right(0)
# 训练模型
model = PINN()
optimizer = torch.optim.Adam(model.parameters(), lr=0.001)
losses = train(model, x_left, x_right, x_inner, y_left, y_right, optimizer, epochs=10000)
# 绘制真实解和预测解的等值线图
x = torch.linspace(0, 1, 100)[:, None]
t = torch.linspace(0, 2, 100)[:, None]
u_true = torch.zeros(100, 100)
u_pred = torch.zeros(100, 100)
for i in range(100):
for j in range(100):
u_true[i, j] = h(g_left(t[j])*(x[i] <= 0.25) + g_right(t[j])*(x[i] >= 0.75) + (1 - x[i])*(x[i] > 0.25)*(x[i] < 0.5)*(t[j] <= 1) + x[i]*(x[i] > 0.5)*(x[i] < 0.75)*(t[j] <= 1) + (1 - x[i])*(x[i] > 0.25)*(x[i] < 0.5)*(t[j] > 1) + (1 - x[i])*(x[i] > 0.5)*(x[i] < 0.75)*(t[j] > 1))
u_pred[i, j] = model(torch.cat([x[i, None], t[j, None]], dim=1)).item()
fig, axs = plt.subplots(1, 3, figsize=(12, 4))
axs[0].contourf(x, t, u_true, levels=100, cmap="rainbow")
axs[0].set_xlabel("x")
axs[0].set_ylabel("t")
axs[0].set_title("True Solution")
axs[1].contourf(x, t, u_pred, levels=100, cmap="rainbow")
axs[1].set_xlabel("x")
axs[1].set_ylabel("t")
axs[1].set_title("Predicted Solution")
axs[2].contourf(x, t, u_true - u_pred, levels=100, cmap="rainbow")
axs[2].set_xlabel("x")
axs[2].set_ylabel("t")
axs[2].set_title("Error")
plt.show()
```
请注意,这段代码假设你已经安装了PyTorch和Matplotlib。
yolov5s核心代码及核心代码解释
以下是 YOLOv5s 的核心代码及其解释:
```
class Detect(nn.Module):
stride = None # strides computed during build
export = False # onnx export
def __init__(self, nc=80, anchors=(), ch=()):
super(Detect, self).__init__()
self.nc = nc # number of classes
self.no = nc + 5 # number of outputs per anchor
self.nl = len(anchors) # number of detection layers
self.na = len(anchors[0]) // 2 # number of anchors
self.grid = [torch.zeros(1)] * self.nl # init grid
a = torch.tensor(anchors).float().view(self.nl, -1, 2)
self.register_buffer('anchors', a)
self.register_buffer('anchor_grid', a.clone().view(self.nl, 1, -1, 1, 1, 2))
self.m = nn.ModuleList(nn.Conv2d(x, self.no * self.na, 1) for x in ch) # output conv
def forward(self, x):
# x(bs,channels,width,height) -> y(bs,anchors,nc+5,width,height)
z = [] # inference output
for i in range(self.nl):
x[i] = self.m[i](x[i]) # conv
bs, _, ny, nx = x[i].shape # x(bs,anchors*(nc+5),width,height) to x(bs,anchors,nc+5,width,height)
x[i] = x[i].view(bs, self.na, self.no, ny, nx).permute(0, 1, 3, 4, 2).contiguous()
if not self.training:
if self.grid[i].shape[2:4] != x[i].shape[2:4]:
self.grid[i] = self._make_grid(nx, ny).to(x[i].device)
y = x[i].sigmoid()
y[..., 0:2] = (y[..., 0:2] * 2. - 0.5 + self.grid[i]) * self.stride[i] # xy
y[..., 2:4] = (y[..., 2:4] * 2) ** 2 * self.anchor_grid[i] # wh
z.append(y.view(bs, -1, self.no))
return x if self.training else (torch.cat(z, 1), x)
def _make_grid(self, nx=20, ny=20):
xv, yv = torch.meshgrid([torch.arange(ny), torch.arange(nx)])
return torch.stack((xv, yv), 2).view((1, 1, ny, nx, 2)).float()
def _initialize_biases(self, cf):
# cf is class frequency
pi = 3.141592653589793
b = [-4.0, -3.0, -2.0, -1.0, 0, 1.0, 2.0, 3.0]
w = [2.0 ** i for i in b]
x = [w[i] * pi / 2 for i in range(len(w))]
y = [(1 - cf[i] / sum(cf)) / (self.nc - 1) for i in range(self.nc)]
for j in range(self.nl):
bias = self.m[j].bias.view(self.na, -1) # conv.bias(255) to (3,85)
if bias.shape[1] == self.no:
# yolov3-tiny
# https://github.com/pjreddie/darknet/blob/master/cfg/yolov3-tiny.cfg
bias[:, 4] += math.log(8. / (640. / 13) ** 2) # obj (8 objects per 13x13 image)
bias[:, 5:] += math.log(0.6 / (self.nc - 0.99)) # cls
elif bias.shape[1] == self.no + 1:
# yolov3 and yolov5
# https://github.com/pjreddie/darknet/blob/master/cfg/yolov3.cfg
# https://github.com/ultralytics/yolov5/blob/master/models/yolo.py
bias[:, 4] += math.log(8. / (640. / 32) ** 2) # obj (8 objects per 32x32 feature map)
bias[:, 5:-1] += math.log(0.6 / (self.nc - 0.99)) # cls
bias[:, -1] += -math.log(1. / (self.na * 0.05)) # ratio
for i in range(self.na):
# if self.na == 1:
# b = bias
# else:
# b = bias[i:i+1]
b = bias[i:i+1]
b[:, 0] += x # x
b[:, 1] += y * 2*pi # y
b[:, 2] += x # w
b[:, 3] += y * 2*pi # h
bias[i:i+1] = b
self.m[j].bias = torch.nn.Parameter(bias.view(-1), requires_grad=True)
```
这个代码实现了 YOLOv5s 中的检测模块,它的主要作用是将输入的特征图进行处理,输出包含物体类别、位置、置信度等信息的检测结果。
- `nc`: 表示物体的类别数;
- `anchors`: 表示锚框的大小和位置;
- `ch`: 表示特征图的通道数;
- `self.grid`: 表示特征图上的网格;
- `self.na`: 表示锚框的数量;
- `y`: 表示检测结果。
在 forward 函数中,首先将输入的特征图通过卷积层进行卷积操作,然后对卷积结果进行变形,得到检测结果。在训练时,会输出特征图,而在测试时,会输出检测结果。
_make_grid 函数将特征图上的每个点映射到原图上,用于计算物体的位置。
_initialize_biases 函数用于初始化偏置,以便更好地检测不同类别的物体。
阅读全文
相关推荐
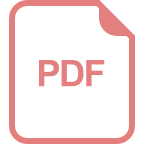
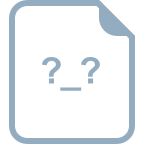


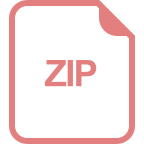
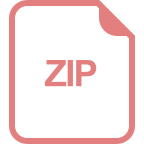
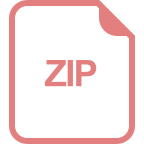
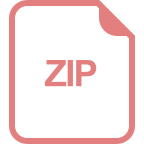
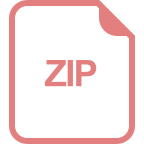
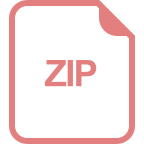