库生成一段c#代码:这段代码首先从源文件夹开始,查找所有二级及以下子文件夹中的.rar文件。然后对每一个找到的压缩文件,解压到目标文件夹,并将解压后的文件命名为对应二级文件夹的名字。
时间: 2024-12-20 19:14:50 浏览: 9
在C#中,你可以使用`System.IO`和`System.IO.Compression`命名空间来完成这样的操作。以下是一段基本的代码示例,用于遍历指定源文件夹及其子文件夹,查找`.rar`文件并进行解压:
```csharp
using System;
using System.IO;
using System.IO.Compression;
class RarFileUnpacker
{
static void Main(string[] args)
{
// 源文件夹路径
string sourceFolderPath = @"C:\SourceFolder";
// 目标文件夹路径
string destinationFolderPath = @"C:\DestinationFolder";
UnzipRarFiles(sourceFolderPath, destinationFolderPath);
}
static void UnzipRarFiles(string folderPath, string destinationPath)
{
if (!Directory.Exists(folderPath))
throw new DirectoryNotFoundException($"Source folder not found at {folderPath}");
foreach (string filePath in Directory.GetFiles(folderPath, "*.rar", SearchOption.AllDirectories))
{
try
{
using (ZipArchive archive = ZipFile.OpenRead(filePath))
{
string subdirName = Path.GetDirectoryName(filePath).Replace(folderPath + "\\", "");
string extractTo = Path.Combine(destinationPath, subdirName);
if (!Directory.Exists(extractTo))
Directory.CreateDirectory(extractTo);
foreach (ZipArchiveEntry entry in archive.Entries)
{
string entryFileName = entry.FullName.Replace(filePath, "");
entry.ExtractToFile(Path.Combine(extractTo, entryFileName), true);
}
}
Console.WriteLine($"{filePath} has been successfully extracted to {extractTo}");
}
catch (Exception ex)
{
Console.WriteLine($"Error occurred while processing file {filePath}: {ex.Message}");
}
}
}
}
```
这个程序首先检查源文件夹是否存在,然后递归地搜索所有二级及以下子目录中的`.rar`文件。对于每个找到的文件,它会创建一个新的目录(如果尚未存在)并将解压后的文件放在该目录下,文件名基于原rar文件所在的子目录名称。
请注意,这个代码假设RAR文件是可以直接打开的,并且不需要密码或特殊权限。如果实际项目中存在这些复杂情况,可能需要添加额外的错误处理和验证步骤。
阅读全文
相关推荐
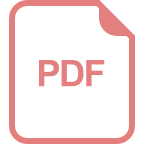
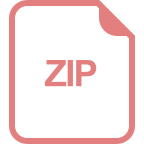
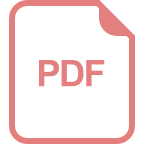















