python可视化高频词词云
时间: 2023-12-22 10:30:05 浏览: 197
以下是使用Python进行可视化高频词词云的示例代码:
```python
from wordcloud import WordCloud
import matplotlib.pyplot as plt
# 假设你已经得到了高频词列表,命名为top_words
top_words = ['词1', '词2', '词3', '词4', '词5', '词6', '词7', '词8', '词9', '词10']
# 将高频词列表转换为字符串
words_str = ' '.join(top_words)
# 创建词云对象
wordcloud = WordCloud(width=800, height=400, background_color='white').generate(words_str)
# 绘制词云图
plt.figure(figsize=(10, 5))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
```
这段代码使用了`WordCloud`库来生成词云图。首先,将高频词列表转换为字符串,然后创建一个词云对象,并指定词云图的宽度、高度和背景颜色。最后,使用`imshow`函数绘制词云图,并使用`axis('off')`函数隐藏坐标轴。运行代码后,将显示生成的词云图。
相关问题
对txt文件进行高频词汇可视化显示 生成词云图
您可以使用Python中的第三方库`wordcloud`来生成词云图。以下是一个简单的示例代码:
```python
import jieba
from wordcloud import WordCloud
import matplotlib.pyplot as plt
# 读取txt文件
with open('example.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 使用jieba分词
words = jieba.lcut(text)
# 去掉停用词
stopwords = {'的', '在', '了', '是', '和', '也', '就', '等'}
words = [word for word in words if word not in stopwords]
# 将分词结果转换成字符串
text = ' '.join(words)
# 生成词云图
wordcloud = WordCloud(background_color='white',
width=800, height=600,
font_path='simhei.ttf').generate(text)
# 显示词云图
plt.imshow(wordcloud)
plt.axis('off')
plt.show()
```
在这个示例代码中,我们使用`jieba`对文本进行分词,并去掉了一些停用词。然后,使用`wordcloud`生成词云图,并使用`matplotlib`显示出来。
请注意,这个示例代码仅仅是一个基本的例子,你可以根据自己的需要进行修改和优化。
打卡桌面中的22012515文件夹,读取其中的文档,将高频词用wordcloud可视化为词云,用python语言
好的,首先需要安装一些必要的库,包括`jieba`用于中文分词和`wordcloud`用于生成词云图。
```python
!pip install jieba
!pip install wordcloud
```
然后我们可以开始读取文件并进行分词,计算高频词。
```python
import os
import jieba
from collections import Counter
from wordcloud import WordCloud
import matplotlib.pyplot as plt
# 读取文件夹中所有的文档
folder_path = '22012515'
file_list = os.listdir(folder_path)
doc_list = []
for file_name in file_list:
file_path = os.path.join(folder_path, file_name)
with open(file_path, 'r', encoding='utf-8') as f:
doc_list.append(f.read())
# 对所有文档进行分词,统计高频词
word_count = Counter()
for doc in doc_list:
words = jieba.cut(doc)
for word in words:
if len(word) > 1: # 只统计长度大于1的词语
word_count[word] += 1
# 获取前50个高频词
top50_words = word_count.most_common(50)
```
接下来,我们可以将高频词可视化为词云。
```python
# 生成词云图
wordcloud = WordCloud(font_path='simhei.ttf', background_color='white', width=800, height=600)
wordcloud.generate_from_frequencies(dict(top50_words))
# 显示词云图
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
```
这样就可以生成一个高频词的词云图了。你可以根据需要调整字体、背景颜色、词云图大小等参数。
完整的代码如下:
```python
import os
import jieba
from collections import Counter
from wordcloud import WordCloud
import matplotlib.pyplot as plt
# 读取文件夹中所有的文档
folder_path = '22012515'
file_list = os.listdir(folder_path)
doc_list = []
for file_name in file_list:
file_path = os.path.join(folder_path, file_name)
with open(file_path, 'r', encoding='utf-8') as f:
doc_list.append(f.read())
# 对所有文档进行分词,统计高频词
word_count = Counter()
for doc in doc_list:
words = jieba.cut(doc)
for word in words:
if len(word) > 1: # 只统计长度大于1的词语
word_count[word] += 1
# 获取前50个高频词
top50_words = word_count.most_common(50)
# 生成词云图
wordcloud = WordCloud(font_path='simhei.ttf', background_color='white', width=800, height=600)
wordcloud.generate_from_frequencies(dict(top50_words))
# 显示词云图
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
```
阅读全文
相关推荐
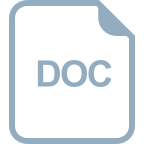
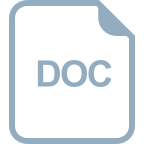
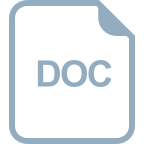
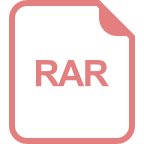
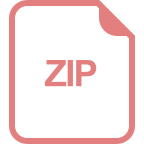
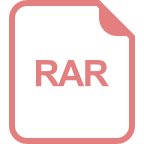
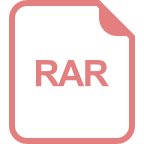
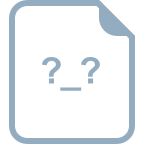
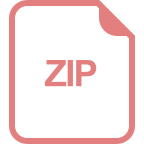
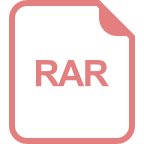
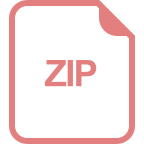
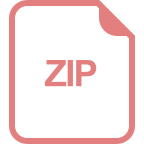
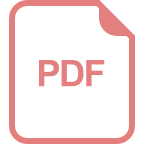
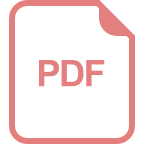
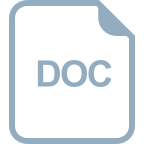
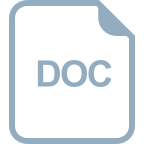
