python实现定义一个包含图书信息(书号、书名、价格)的链表
时间: 2024-10-07 20:04:19 浏览: 50
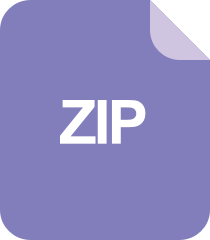
使用 python3 实现一个链表
在Python中,我们可以使用类和结构化数据来创建一个简单的链表来存储图书信息。首先,我们需要定义一个Book类来表示单本书,包含书号、书名和价格属性。然后,我们将创建一个Node类,用于链表节点,每个节点都包含一个Book对象以及指向下一个节点的引用。这里是一个简单的实现:
```python
class Book:
def __init__(self, isbn, title, price):
self.isbn = isbn
self.title = title
self.price = price
class Node:
def __init__(self, book, next=None):
self.book = book
self.next = next
class LibraryList:
def __init__(self):
self.head = None
def add_book(self, isbn, title, price):
new_node = Node(Book(isbn, title, price))
if not self.head:
self.head = new_node
else:
current = self.head
while current.next:
current = current.next
current.next = new_node
def display_books(self):
current = self.head
while current:
print(f"ISBN: {current.book.isbn}, Title: {current.book.title}, Price: {current.book.price}")
current = current.next
# 使用示例
library_list = LibraryList()
library_list.add_book(1001, "Python Crash Course", 49.99)
library_list.add_book(1002, "Clean Code", 27.99)
library_list.display_books()
```
在这个例子中,`LibraryList`类维护了一个头节点,并提供添加新书和显示所有书籍的方法。
阅读全文
相关推荐
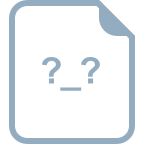
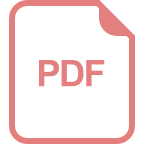










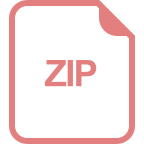
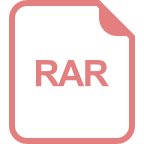
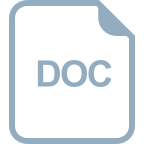
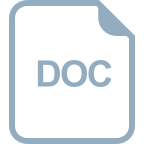
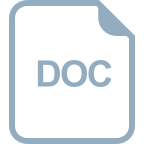
