在Java编写同一个功能的应用程序,实现下列功能: 输入20名同学的学号、姓名、三门课程成绩; 计算每位同学的总分与平均分; 输出成绩表、显示总分最高分与最低分学生的成绩信息。
时间: 2024-10-22 20:21:55 浏览: 48
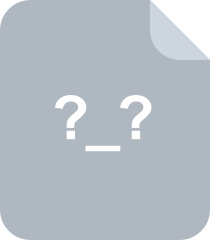
课程设计二: 题目: 学生成绩管理系统 功能: 实现学生信息管理 包括:录入、查询、排序等功能 C语言
在Java中,你可以创建一个学生类(Student),包含学号(id)、姓名(name)和三门课程的成绩(scores[])。然后利用数组或列表来存储20位同学的信息。接下来是一个简单的示例说明如何实现这个功能:
```java
import java.util.ArrayList;
import java.util.Scanner;
class Student {
int id;
String name;
double scores[];
// 构造函数
public Student(int id, String name) {
this.id = id;
this.name = name;
this.scores = new double[3]; // 假设每门课满分100分
}
// 计算总分和平均分
double calculateTotalScore() {
double total = 0;
for (double score : scores) {
total += score;
}
return total / scores.length;
}
}
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
ArrayList<Student> students = new ArrayList<>();
// 输入学生信息
for (int i = 1; i <= 20; i++) {
System.out.println("请输入第" + i + "位同学的信息:");
Scanner input = new Scanner(scanner.nextLine());
int id = input.nextInt();
String name = input.next();
double[] scores = {input.nextDouble(), input.nextDouble(), input.nextDouble()};
students.add(new Student(id, name, scores));
}
// 计算总分和平均分并输出成绩表
System.out.println("\n成绩表:");
for (Student student : students) {
double totalScore = student.calculateTotalScore();
System.out.printf("学号: %d, 姓名: %s, 总分: %.2f, 平均分: %.2f\n",
student.id, student.name, totalScore, totalScore);
}
// 找出最高分和最低分的学生
double maxScore = students.get(0).calculateTotalScore();
double minScore = maxScore;
Student maxStudent = null;
Student minStudent = null;
for (Student s : students) {
double score = s.calculateTotalScore();
if (score > maxScore) {
maxScore = score;
maxStudent = s;
} else if (score < minScore) {
minScore = score;
minStudent = s;
}
}
System.out.printf("\n最高分学生信息: 学号: %d, 姓名: %s, 成绩: %.2f%n",
maxStudent.id, maxStudent.name, maxScore);
System.out.printf("最低分学生信息: 学号: %d, 姓名: %s, 成绩: %.2f%n",
minStudent.id, minStudent.name, minScore);
scanner.close();
}
}
```
阅读全文
相关推荐
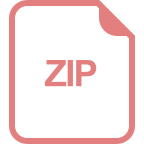
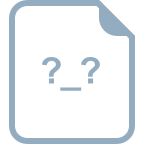
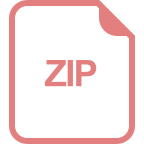
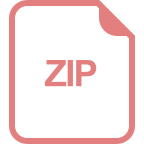
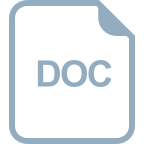
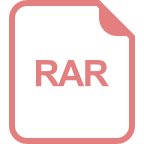
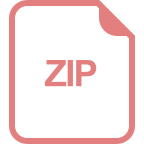
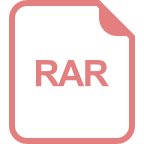
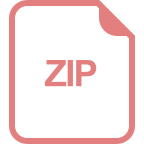
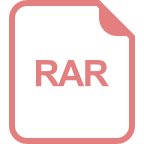
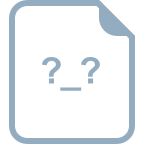
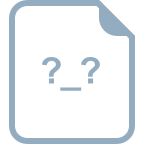
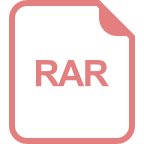
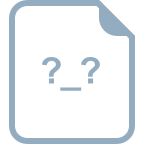
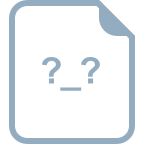
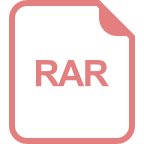
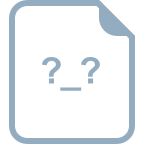