汉诺塔代码,包含输出“请输入盘子个数”
时间: 2024-11-12 21:40:53 浏览: 25
汉诺塔是一个经典的递归问题,通常涉及三个柱子(A、B 和 C)和一些圆盘,目标是从柱子 A 移动所有的圆盘到柱子 C,过程中始终遵守两个规则:只能移动一个圆盘,且任何时候都不能让大盘子放在小盘子上面。
以下是使用Python编写的一个简单版汉诺塔游戏,它首先提示用户输入圆盘的数量,然后递归地执行移动过程:
```python
def hanoi(n, source, auxiliary, target):
if n > 0:
# 将前n-1个圆盘从源柱子移动到辅助柱子,再移到目标柱子
hanoi(n - 1, source, target, auxiliary)
# 把最大的圆盘直接从源柱子移到目标柱子
print(f"Move disk {n} from rod {source} to rod {target}")
# 最后将前n-1个圆盘从辅助柱子移动到目标柱子,覆盖之前的大盘子
hanoi(n - 1, auxiliary, source, target)
# 主函数,提示用户输入盘子数量并调用hanoi函数
if __name__ == "__main__":
print("请输入盘子个数:")
num_disks = int(input())
hanoi(num_disks, 'A', 'B', 'C')
```
当运行这个程序时,用户会被问及盘子数量,然后按照正确的步骤看到汉诺塔游戏的模拟。
相关问题
汉诺塔c语言 要求由用户输入盘子数和柱子名称
好的,以下是汉诺塔的 C 语言代码,可以由用户输入盘子数和柱子名称:
```c
#include <stdio.h>
void hanoi(int n, char from, char to, char via) {
if (n == 1) {
printf("Move disk from %c to %c\n", from, to);
return;
}
hanoi(n-1, from, via, to);
printf("Move disk from %c to %c\n", from, to);
hanoi(n-1, via, to, from);
}
int main() {
int n;
char a, b, c;
printf("Enter the number of disks: ");
scanf("%d", &n);
printf("Enter the name of first pole: ");
scanf(" %c", &a);
printf("Enter the name of second pole: ");
scanf(" %c", &b);
printf("Enter the name of third pole: ");
scanf(" %c", &c);
hanoi(n, a, c, b);
return 0;
}
```
在这个程序中,我们使用递归来解决汉诺塔问题。`hanoi` 函数接受三个参数:盘子数量 `n`,起始柱子名称 `from`,目标柱子名称 `to`,以及中介柱子名称 `via`。如果只有一个盘子,我们直接将它从起始柱子移动到目标柱子。否则,我们将前 n-1 个盘子从起始柱子移动到中介柱子,然后将第 n 个盘子从起始柱子移动到目标柱子,最后将前 n-1 个盘子从中介柱子移动到目标柱子。
在 `main` 函数中,我们首先要求用户输入盘子数量和三个柱子的名称,然后调用 `hanoi` 函数来解决问题。
编写程序实现汉诺塔,用户输入盘子个数,输出盘子移动的信息
好的,这个问题很有趣!以下是汉诺塔的程序实现:
```
def hanoi(n, source, target, auxiliary):
if n == 1:
print("Move disk 1 from source", source, "to target", target)
return
hanoi(n-1, source, auxiliary, target)
print("Move disk", n, "from source", source, "to target", target)
hanoi(n-1, auxiliary, target, source)
n = int(input("Enter number of disks: "))
hanoi(n, 'A', 'C', 'B')
```
这个程序会要求用户输入盘子的个数,然后输出每一步移动的信息,实现汉诺塔的移动。希望这个程序对你有帮助!
阅读全文
相关推荐
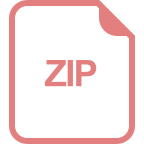
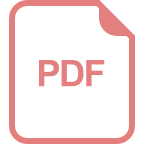
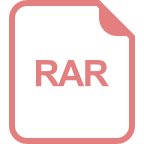













