写一个贪吃蛇小游戏,用Python
时间: 2024-06-15 22:01:47 浏览: 19
创建一个简单的贪吃蛇游戏,你可以使用Python的pygame库,这是一个强大的游戏开发框架。以下是编写一个基础贪吃蛇游戏的基本步骤:
1. 安装pygame库:
```shell
pip install pygame
```
2. 导入所需模块和设置窗口:
```python
import pygame
pygame.init()
screen_width, screen_height = 800, 600
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("贪吃蛇")
```
3. 创建游戏元素(贪吃蛇、食物和得分):
```python
snake_block_size = 10
snake = [(50, 50), (40, 50), (30, 50)] # 初始位置和身体部分
food = (70, 70) # 食物初始位置
score = 0
```
4. 游戏循环和更新逻辑:
```python
clock = pygame.time.Clock()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
keys = pygame.key.get_pressed()
if keys[pygame.K_UP] and snake > 0: # 上箭头移动
snake.insert(0, (snake, snake - snake_block_size))
elif keys[pygame.K_DOWN] and snake < screen_height - snake_block_size: # 下箭头
snake.insert(0, (snake, snake + snake_block_size))
# 其他方向键类似
if snake == food:
score += 1
food = (random.randint(0, screen_width // snake_block_size) * snake_block_size,
random.randint(0, screen_height // snake_block_size) * snake_block_size)
snake.append((0, 0)) # 增加新蛇段
# 移除蛇尾
snake.pop()
# 检查碰撞
for body_part in snake[1:]:
if body_part == snake:
pygame.quit()
quit()
screen.fill((0, 0, 0)) # 清屏
for body_part in snake:
pygame.draw.rect(screen, (255, 0, 0), pygame.Rect(body_part, (snake_block_size, snake_block_size)))
pygame.draw.rect(screen, (0, 255, 0), pygame.Rect(food, (snake_block_size, snake_block_size)))
text = f"Score: {score}"
font = pygame.font.SysFont(None, 30)
text_surface = font.render(text, True, (255, 255, 255))
screen.blit(text_surface, (10, 10))
pygame.display.update()
clock.tick(10) # 控制帧率
```
5. 结束游戏:
游戏结束后,记得添加`pygame.quit()`和`quit()`以关闭窗口。
相关推荐
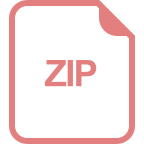
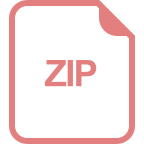
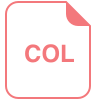
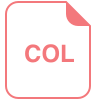
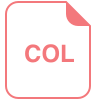
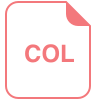
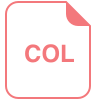




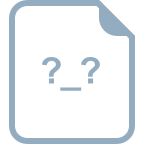
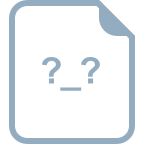
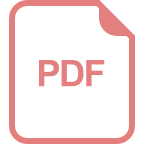
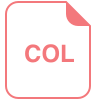
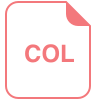