ssh 多线程python代码
时间: 2024-04-28 18:16:34 浏览: 12
SSH(Secure Shell)是一种网络协议,用于在不安全的网络上安全地进行远程登录和执行命令。在Python中,可以使用paramiko库来实现SSH连接和执行命令的功能。下面是一个使用多线程实现SSH连接和执行命令的Python代码示例:
```python
import paramiko
import threading
def ssh_command(ip, username, password, command):
client = paramiko.SSHClient()
client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
client.connect(ip, username=username, password=password)
stdin, stdout, stderr = client.exec_command(command)
print(f"Output from {ip}:")
for line in stdout:
print(line.strip())
client.close()
# 定义要连接的主机列表
hosts = [
{"ip": "192.168.1.1", "username": "user1", "password": "password1"},
{"ip": "192.168.1.2", "username": "user2", "password": "password2"},
# 添加更多主机...
]
# 定义要执行的命令
command = "ls -l"
# 创建线程列表
threads = []
# 创建并启动线程
for host in hosts:
t = threading.Thread(target=ssh_command, args=(host["ip"], host["username"], host["password"], command))
t.start()
threads.append(t)
# 等待所有线程执行完毕
for t in threads:
t.join()
```
上述代码中,我们首先导入了paramiko库,然后定义了一个`ssh_command`函数,该函数用于建立SSH连接并执行命令。接下来,我们定义了要连接的主机列表和要执行的命令。然后,我们创建了一个线程列表,并使用多线程的方式分别连接每个主机并执行命令。最后,我们等待所有线程执行完毕。
相关推荐
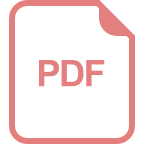














