C++ 线程池 传递多个参数 执行多线程
时间: 2024-09-07 09:04:22 浏览: 46
在C++中,如果你想要使用线程池来同时执行多个带参数的任务,你可以利用`std::packaged_task`配合`std::async`或者自定义`ThreadFunctionRunner`类。这里有一个简单示例,展示如何传递多个参数到线程池中的任务:
```cpp
#include <iostream>
#include <thread>
#include <vector>
#include <future>
#include <tuple>
// 创建一个接受两个参数的包装函数
template<typename Func, typename... Args>
std::packaged_task<decltype(Func(Args...))()> create_task(Func func, Args... args) {
return std::packaged_task<decltype(Func(Args...))()>([func = std::move(func), args = std::make_tuple(std::forward<Args>(args)...)]() mutable {
return func(args...);
});
}
class ThreadPool {
private:
std::vector<std::future<void>> futures;
const size_t max_threads;
public:
ThreadPool(size_t num_threads = std::thread::hardware_concurrency()) : max_threads(num_threads) {}
template<typename Func, typename... Args>
void enqueue(Func func, Args... args) {
if (futures.size() < max_threads) {
futures.push_back(create_task(func, args...).get_future());
execute();
} else {
std::cout << "ThreadPool is full, task will wait until slots are available." << std::endl;
}
}
void join_all() {
for (auto& future : futures) {
future.wait(); // 等待所有任务完成
}
futures.clear();
}
private:
void execute() {
if (!futures.empty()) {
auto task = futures.front().get_future();
futures.erase(futures.begin());
std::thread worker([task] {
task.wait(); // 当前线程执行任务
});
worker.join(); // 等待线程结束
}
}
};
int main() {
ThreadPool pool;
for (size_t i = 0; i < 5; ++i) {
pool.enqueue([](int a, int b) {
std::cout << "Task " << i << " executed with arguments (" << a << ", " << b << ")\n";
}, i, i * 10);
}
pool.join_all(); // 等待所有任务完成
return 0;
}
```
在这个示例中,`enqueue`方法接受一个函数`Func`和任意数量的参数`Args`,创建一个包装后的`packaged_task`,并将任务和未来结果存储在`futures`向量中。当线程池有足够的空间时,它会开始执行新的任务。
阅读全文
相关推荐
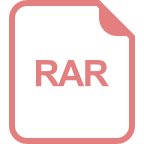
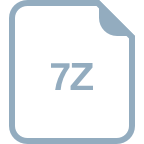
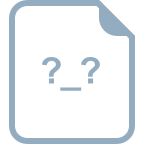

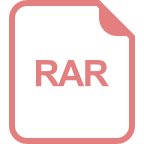
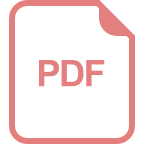
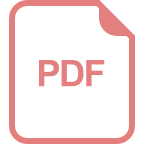
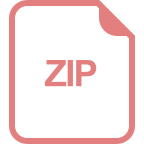
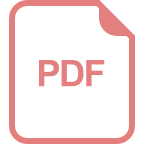
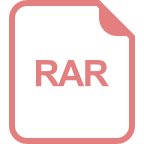
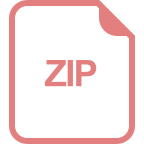
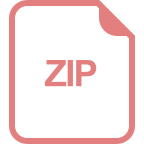
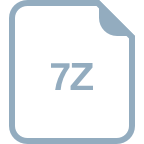
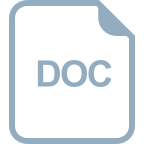
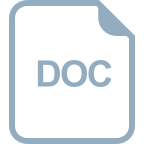
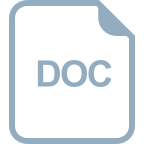
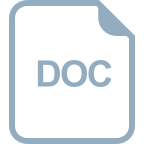
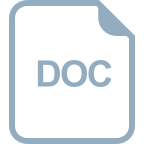
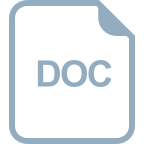