如何使用C语言实现一个基本的队列结构,并演示其功能,包括将整数1, 2, 3依次进行入队(enqueue)操作,然后按照先进先出(FIFO)原则执行一次出队(dequeue)操作?
时间: 2024-10-22 07:27:12 浏览: 45
在C语言中,我们可以使用数组和两个指针(一个指向队首,一个指向队尾)来实现一个基本的顺序队列。以下是一个简单的示例:
```c
#include <stdio.h>
#define MAX_SIZE 5
int queue[MAX_SIZE]; // 定义队列
int front = -1; // 队头指针
int rear = -1; // 队尾指针
// 入队函数
void enqueue(int value) {
if ((rear + 1) % MAX_SIZE == front) { // 当队满时
printf("Error: Queue is full.\n");
return;
}
rear = (rear + 1) % MAX_SIZE; // 后进先出,更新队尾
queue[rear] = value;
printf("Enqueue %d at position %d\n", value, rear);
}
// 出队函数
int dequeue() {
if (front == -1) { // 队空
printf("Error: Queue is empty.\n");
return -1;
}
int removed_value = queue[front]; // 取出队头元素
queue[front] = -1; // 清空队头位置
front = (front + 1) % MAX_SIZE; // 更新队头指针
printf("Dequeue %d from position %d\n", removed_value, front);
return removed_value;
}
int main() {
// 初始化队列为空
while(front != rear) {
enqueue(-1);
}
// 入队1, 2, 3
enqueue(1);
enqueue(2);
enqueue(3);
// 出队并打印
int dequeued_value = dequeue();
printf("First element dequeued: %d\n", dequeued_value);
return 0;
}
```
在这个程序中,我们首先初始化队列为空,然后分别进行1, 2, 3的入队操作。接着尝试出队一次,由于刚刚入了三个元素,所以会出队第一个元素1。
阅读全文
相关推荐
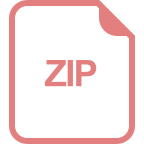
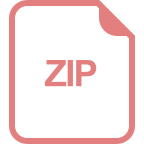
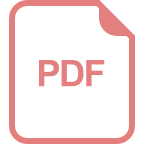
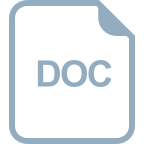
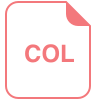













