用c++设计一个单链表结构,该链表结构数据域存储整数。
时间: 2024-09-26 09:04:28 浏览: 33
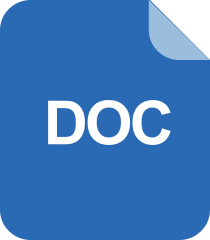
数据结构--单链表操作--实验报告.doc

在C++中,我们可以使用结构体(struct)和指针来设计一个简单的单链表结构,其中每个节点包含一个整数值。下面是一个基本的单链表节点(Node)和链表(LinkedList)的实现:
```cpp
// 定义单链表节点结构体
struct ListNode {
int data; // 数据域存储整数
ListNode* next; // 指向下一个节点的指针
};
// 单链表类
class LinkedList {
private:
ListNode* head; // 链表头节点
public:
// 构造函数,初始化空链表
LinkedList() : head(nullptr) {}
// 插入元素到链表头部
void insert(int value) {
ListNode* newNode = new ListNode();
newNode->data = value;
newNode->next = head;
head = newNode;
}
// 查找指定值的节点
ListNode* search(int value) const {
ListNode* current = head;
while (current != nullptr && current->data != value) {
current = current->next;
}
return current;
}
// 删除指定值的节点
void remove(int value) {
if (head == nullptr || head->data != value) return;
ListNode* temp = head;
head = head->next;
delete temp;
}
// 打印链表所有节点
void printList() const {
ListNode* current = head;
while (current != nullptr) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << std::endl;
}
};
```
阅读全文
相关推荐



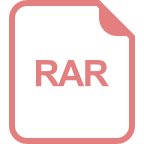
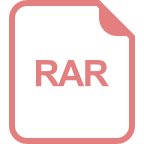
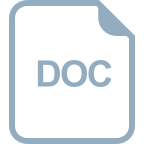







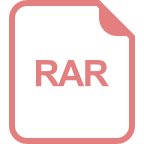
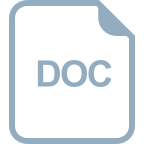
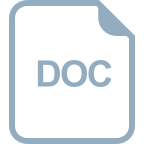


