stack的基本操作c++
时间: 2024-09-18 16:16:51 浏览: 29
栈是一种线性的数据结构,它遵循“先进后出”(Last In First Out,LIFO)的原则。在C++中,你可以使用标准库中的`std::stack`容器来实现基本的操作。以下是`std::stack`的一些常用操作:
1. **创建栈**:
```cpp
#include <stack>
std::stack<int> s;
```
这里创建了一个空的整数栈。
2. **元素入栈(push)**:
```cpp
s.push(5); // 将5压入栈顶
```
3. **元素出栈(pop)**:
```cpp
int topValue = s.top(); // 获取栈顶元素
s.pop(); // 删除并返回栈顶元素(这里是5)
```
4. **查看栈顶元素(top)**:
```cpp
int value = s.top(); // 返回栈顶元素,但不删除
```
5. **判断是否为空(empty)**:
```cpp
bool isEmpty = s.empty(); // 如果栈为空,返回true;否则返回false
```
6. **获取大小(size)**:
```cpp
size_t size = s.size(); // 返回栈中元素的数量
```
7. **清空栈(clear)**:
```cpp
s.clear(); // 清除所有元素
```
相关问题
栈的基本操作c++ 创建
栈是一种线性的数据结构,其特点是只能在一端(称为栈顶)进行插入和删除操作,即后进先出(Last In First Out, LIFO)。在C++中,我们可以使用标准库中的`std::stack`容器来实现基本的栈操作。
创建一个空栈并使用`std::stack`的基本步骤如下:
```cpp
#include <iostream>
#include <stack>
int main() {
// 创建一个名为myStack的栈实例
std::stack<int> myStack;
// 操作1:检查栈是否为空
if (myStack.empty()) {
std::cout << "栈是空的.\n";
}
// 操作2:压入元素(入栈)
myStack.push(10); // myStack现在包含10
myStack.push(20);
// 操作3:查看栈顶元素
int topElement = myStack.top(); // 返回但不移除栈顶元素
std::cout << "栈顶元素是: " << topElement << "\n";
// 操作4:弹出元素(出栈)
myStack.pop(); // 移除并返回栈顶元素(现在栈中只有20)
// 操作5:再次检查栈是否为空
if (myStack.empty()) {
std::cout << "栈已经为空.\n";
}
return 0;
}
```
C++中stack的基本函数
C++中stack是一个容器适配器,通过在内部使用deque或list来实现栈的基本操作。以下是stack的基本函数:
1. push():将元素压入栈顶。
2. pop():将栈顶元素弹出。
3. top():返回栈顶元素。
4. empty():判断栈是否为空,如果为空返回true,否则返回false。
5. size():返回栈中元素的个数。
以下是一个简单的stack示例:
```c++
#include <iostream>
#include <stack>
using namespace std;
int main() {
stack<int> s;
s.push(1);
s.push(2);
s.push(3);
while (!s.empty()) {
cout << s.top() << " ";
s.pop();
}
return 0;
}
```
输出结果为:
```
3 2 1
```
阅读全文
相关推荐
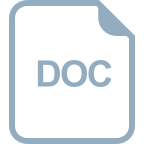
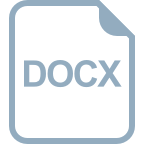
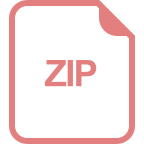













