请用顺序线性表实现约瑟夫环问题写一个完整详细的代码,要求用c++
时间: 2024-10-07 17:06:04 浏览: 74
约瑟夫环问题是这样的:给定一个数组(环形结构),数组中的每个元素代表一个人,从第0位开始,按照顺时针方向计数,每次跳过一位,直到有人再次回到起始位置,那个人就获胜。下面是一个使用C++实现约瑟夫环问题的例子:
```cpp
#include <iostream>
using namespace std;
// 定义数组大小
const int ARRAY_SIZE = 10;
// 求解约瑟夫环问题的函数
int josephus(int n, int k) {
// 初始化环中的指针和当前轮数
int pointer = 0;
int rounds = 0;
// 循环直到找到胜利者
while (true) {
// 输出当前轮数的人
cout << "Round " << rounds << ": Player " << pointer << endl;
// 更新指针,跳过k个人
pointer = (pointer + k - 1) % ARRAY_SIZE;
rounds++;
// 如果轮到起点,则找到胜利者并返回其索引
if (pointer == 0)
return pointer;
}
}
int main() {
// 输入人数和每轮跳跃的人数
int numPlayers, jump;
cout << "Enter the number of players and the skip count: ";
cin >> numPlayers >> jump;
// 确保skip count小于或等于numPlayers
if (jump >= numPlayers) {
cout << "Error: Skip count must be less than the number of players." << endl;
return 1;
}
// 调用函数计算结果
int winnerIndex = josephus(numPlayers, jump);
cout << "The winner is player " << winnerIndex << "." << endl;
return 0;
}
```
在这个代码中,`josephus` 函数接受两个参数:数组中的人数 `n` 和每轮跳跃的人数 `k`。它使用一个循环,每次循环后,指针向前移动 `k-1` 步,并增加一轮次数。当指针再次达到0时,表示找到了胜利者。
阅读全文
相关推荐














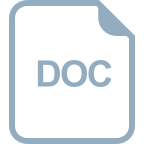



