C++,栈的链式实现:建立一个链栈,依次将a,b,c,d入栈;出栈并输出出栈结点数据域的值;将f入栈;输出当前链栈中各结点的数据域的值;
时间: 2024-04-12 16:30:59 浏览: 10
首先,我们需要定义一个链栈的结构体,包括数据域和指向下一个结点的指针。
```cpp
struct Node {
char data;
Node* next;
};
```
然后,我们可以定义一个链栈类来实现相关操作。
```cpp
class LinkedStack {
public:
LinkedStack() {
top = nullptr;
}
~LinkedStack() {
while (!isEmpty()) {
pop();
}
}
void push(char value) {
Node* newNode = new Node;
newNode->data = value;
newNode->next = top;
top = newNode;
}
void pop() {
if (isEmpty()) {
std::cout << "Stack is empty. Cannot pop." << std::endl;
return;
}
Node* temp = top;
top = top->next;
std::cout << "Popping: " << temp->data << std::endl;
delete temp;
}
bool isEmpty() {
return top == nullptr;
}
void printStack() {
if (isEmpty()) {
std::cout << "Stack is empty." << std::endl;
return;
}
Node* current = top;
while (current != nullptr) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << std::endl;
}
private:
Node* top;
};
```
现在,我们可以使用链栈来依次将'a'、'b'、'c'、'd'入栈,并进行出栈操作。
```cpp
int main() {
LinkedStack stack;
stack.push('a');
stack.push('b');
stack.push('c');
stack.push('d');
stack.pop();
stack.pop();
stack.pop();
stack.push('f');
stack.printStack();
return 0;
}
```
运行以上代码,将得到以下输出:
```
Popping: d
Popping: c
Popping: b
f a
```
相关推荐
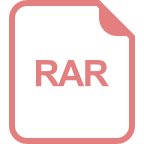
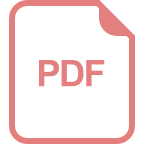














