编写一个 Python 程序,读取一个包含多行文本的文件,每行文本包含 一组单词。要求程序: 1. 统计文件中每个单词出现的频率,并按出现频率从高到低输出前 10 个单 词及其频率。 2. 将出现频率最低的 10 个单词写入一个新的文件。
时间: 2024-09-22 19:02:33 浏览: 53
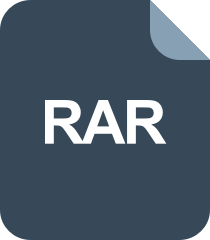
统计文本文件中的字符单词和行数
首先,我们需要编写一个 Python 程序来完成这个任务。我们可以使用内置的 `collections` 模块中的 `Counter` 类来统计单词频率,`open()` 函数来读取文件,以及 `itertools.islice()` 来获取频率最低的单词。下面是一个示例:
```python
import re
from collections import Counter
def read_file_and_count_words(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read().lower() # 转换为小写并读取内容
words = re.findall(r'\b\w+\b', content) # 使用正则表达式提取单词
word_counts = Counter(words)
return word_counts
def top_10_frequent_words(word_counts):
top_10 = word_counts.most_common(10)
print("Top 10 most frequent words:")
for word, freq in top_10:
print(f"{word}: {freq}")
def bottom_10_least_frequent_words(word_counts, output_file_path):
least_common = word_counts.most_common()[:-11:-1] # 获取频率最低的10个单词
with open(output_file_path, 'w', encoding='utf-8') as output_file:
for word, freq in least_common:
output_file.write(f"{word}: {freq}\n")
# 示例文件路径
input_file = "example.txt"
output_file = "bottom_10_words.txt"
word_counts = read_file_and_count_words(input_file)
top_10_frequent_words(word_counts)
bottom_10_least_frequent_words(word_counts, output_file)
阅读全文
相关推荐
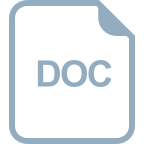
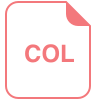
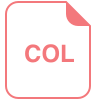
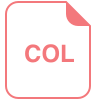
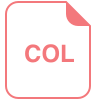
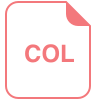
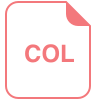
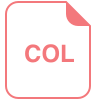
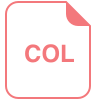
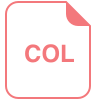
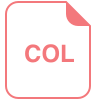
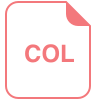
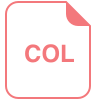
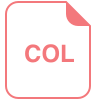
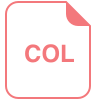
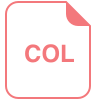
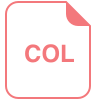
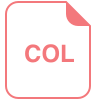