c++实现线性表的建立、插入、删除、查找
时间: 2024-10-08 09:15:42 浏览: 30
在 C++ 中,可以使用多种数据结构来实现线性表,常见的有数组(Array)和链表(Linked List)。这里分别介绍这两种情况下的操作:
**一、数组实现线性表**
1. **建立(初始化)**:
```cpp
int linearList[10]; // 创建一个包含10个元素的整型数组
```
2. **插入**:
- 使用下标可以直接插入元素,前提是有足够的空间:
```cpp
linearList[5] = value; // 插入到第6个位置(数组从0开始计数)
```
3. **删除**:
- 如果删除特定索引处的元素,需要移动其他元素并减小大小:
```cpp
for(int i = 5; i < size - 1; i++) {
linearList[i] = linearList[i + 1];
}
size--; // 缩减数组大小
```
- 注意:数组通常无法直接删除单个元素,除非预先知道新的大小。
4. **查找**:
```cpp
int index = findIndex(linearList, target); // 自己实现查找函数
```
**二、链表实现线性表**
1. **建立(初始化)**:
```cpp
struct Node {
int data;
Node* next;
};
Node* head = nullptr; // 创建空链表
```
2. **插入**:
```cpp
Node* newNode = new Node{value, nullptr};
if(head == nullptr) {
head = newNode;
} else {
Node* current = head;
while(current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
```
3. **删除**:
```cpp
delete head; // 删除头节点
head = head->next;
// 或者遍历链表删除特定值的节点
Node* current = head;
while(current != nullptr && current->data != target) {
current = current->next;
}
if(current != nullptr) {
Node* temp = current->next;
delete current;
current = temp;
}
```
4. **查找**:
```cpp
Node* foundNode = searchLinkedList(head, target);
```
阅读全文
相关推荐
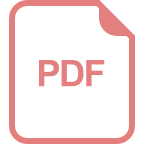
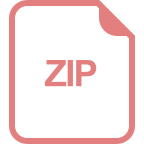
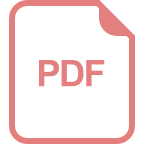
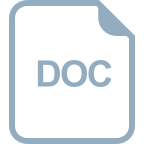
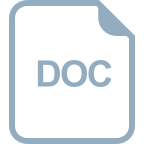
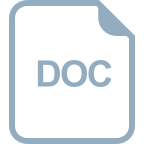
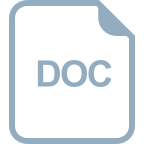
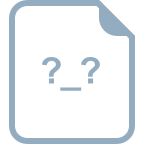
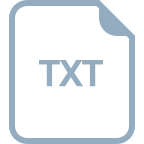
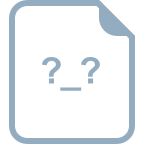
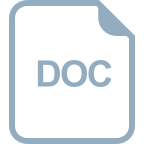
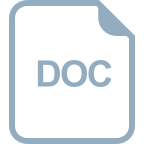
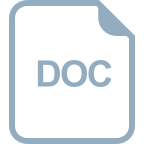
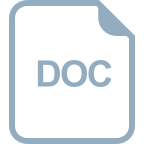
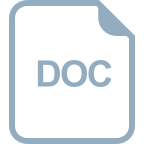
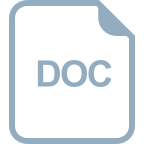
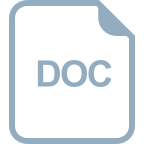

