用C语言设计以下文法的递归下降分析程序,并判断任意输入的符号串是否合法,并能指出输入的字符串哪里不合法。文法规则S->aSe S->B B->bBe B->C C->cCc C->d
时间: 2024-12-25 18:29:46 浏览: 27
在C语言中,我们可以使用递归下降解析器(Recursive Descent Parser)来处理这个文法。首先,我们需要创建几个函数,每个对应文法中的非终结符(S, B, C)。我们将通过函数调用来检查输入串是否符合规则。下面是一个简单的示例:
```c
#include <stdio.h>
#include <stdbool.h>
// 定义文法符号对应的函数
bool is_a(char c) {
return c == 'a';
}
bool is_b(char c) {
return c == 'b';
}
bool is_c(char c) {
return c == 'c' || c == 'd';
}
// 解析器函数
void parse_S(char *input, int* index) {
if (is_a(input[*index])) {
printf("Valid rule: S -> aS\n");
(*index)++;
parse_S(input, index);
parse_B(input, index);
} else if (is_b(input[*index])) {
// 如果当前字符是B,但后续不是e,则表示非法
char next = input[(*index) + 1];
if (!is_e(next)) {
printf("Invalid: expecting 'e' after 'B', but found '%c'\n", next);
return;
}
(*index)++;
parse_B(input, index);
} else {
printf("Invalid: expecting 'a' or 'B', but found '%c'\n", input[*index]);
}
}
void parse_B(char *input, int* index) {
if (is_b(input[*index])) {
printf("Valid rule: B -> bB\n");
(*index)++;
parse_B(input, index);
parse_E(input, index);
} else if (is_c(input[*index])) {
// 同理,对于C,需要检查后面的字符是否匹配
parse_C(input, index);
parse_E(input, index);
} else {
printf("Invalid: expecting 'b' or 'C', but found '%c'\n", input[*index]);
}
}
// 其他类似解析函数,如parse_C...
void parse_E(char *input, int* index) {
if (input[*index] != 'e') {
printf("Invalid: expecting 'e' at the end of B/C, but found '%c'\n", input[*index]);
}
(*index)++;
}
int main() {
char input[] = "abcde"; // 测试输入
int index = 0;
parse_S(input, &index);
if (index == sizeof(input)) {
printf("The input string is valid.\n");
} else {
printf("Not all characters have been processed, check the error messages above.\n");
}
return 0;
}
```
在这个示例中,`parse_S`, `parse_B`, 和 `parse_C` 函数分别处理S、B和C的转换。如果遇到不符合规则的字符,会打印错误信息并停止解析。运行该程序,你可以测试输入字符串是否合法以及在哪一步出现错误。
阅读全文
相关推荐

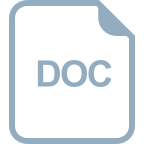



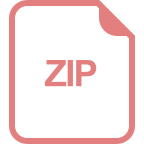












