yolov7 test.py
时间: 2024-02-02 11:02:50 浏览: 32
对不起,我无法运行实际的代码。但是,我可以为您提供关于使用YOLOv7进行目标检测的一般指导。
首先,确保您已经安装了必要的依赖项和库,例如Python、OpenCV和PyTorch。然后,按照以下步骤进行操作:
1. 下载YOLOv7的源代码。您可以在GitHub上找到许多不同版本的实现。
2. 准备数据集和标注文件。您需要一个包含图像和对应标注的数据集,标注通常以XML或JSON格式保存。
3. 配置模型参数。根据您的需求,您可能需要调整模型的配置文件,例如输入图像尺寸、类别数、训练参数等。
4. 进行模型训练。使用数据集和标注文件对模型进行训练。您可以选择从头开始训练,或者使用预训练模型进行迁移学习。
5. 进行测试和推理。在测试阶段,您可以使用训练好的模型对新的图像进行目标检测。编写一个脚本(例如test.py)来加载模型并对图像进行推理。
请注意,以上只是一般步骤,并且可能因YOLOv7实现的不同而有所差异。确保仔细阅读所选实现的文档和示例代码,并根据您的需求进行适当的调整。
相关问题
yolov7 test.py是干嘛的
引用\[1\]:根据你提供的信息,yolov7 test.py是用于测试模型的脚本。在你的情况下,你意识到使用test.py测试数据时出现了问题,因为它使用的是训练时划分的验证集中的数据,而不是你想要的测试数据。你正确地意识到应该使用detect.py来进行测试,因为detect.py中预设了不同形式数据的检测接口,包括视频流、图片地址和视频文件等等。通过使用detect.py,你成功地得到了你想要的结果。\[1\]
问题: yolov7 test.py是干嘛的?
回答: yolov7 test.py是用于测试模型的脚本。它可以用于检测和评估训练好的yolov7模型在给定数据集上的性能。然而,在你的情况下,你意识到使用test.py测试数据时出现了问题,因为它使用的是训练时划分的验证集中的数据,而不是你想要的测试数据。因此,你正确地使用了detect.py来进行测试,并成功地得到了你想要的结果。\[1\]
#### 引用[.reference_title]
- *1* [yoloV7中test.py和train.py的区别](https://blog.csdn.net/weixin_41942424/article/details/125996214)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^koosearch_v1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* *3* [YOLOv7教程系列:一、基于自定义数据集训练专属于自己的目标检测模型(保姆级教程,含数据集预处理),包含...](https://blog.csdn.net/weixin_45921929/article/details/126448031)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^koosearch_v1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
yolov7 test.py详解
YoloV7是目标检测算法YOLO的最新版本,相较于之前的版本,它在模型结构、训练策略和速度等方面都有了较大的改进。test.py文件是用于测试已经训练好的模型的脚本,下面是对test.py文件的详细解释:
1. 导入必要的库和模块
```python
import argparse
import os
import platform
import shutil
import time
from pathlib import Path
import cv2
import torch
import torch.backends.cudnn as cudnn
import numpy as np
from models.experimental import attempt_load
from utils.datasets import LoadStreams, LoadImages
from utils.general import check_img_size, check_requirements, check_imshow, \
non_max_suppression, apply_classifier, scale_coords, xyxy2xywh, strip_optimizer, set_logging
from utils.plots import plot_one_box
from utils.torch_utils import select_device, load_classifier, time_synchronized
```
这里导入了一些必要的库和模块,比如PyTorch、OpenCV、NumPy等,以及用于测试的模型、数据集和一些工具函数。
2. 定义输入参数
```python
parser = argparse.ArgumentParser()
parser.add_argument('--weights', nargs='+', type=str, default='yolov5s.pt', help='model.pt path(s)')
parser.add_argument('--source', type=str, default='data/images', help='source')
parser.add_argument('--img-size', type=int, default=640, help='inference size (pixels)')
parser.add_argument('--conf-thres', type=float, default=0.25, help='object confidence threshold')
parser.add_argument('--iou-thres', type=float, default=0.45, help='IOU threshold for NMS')
parser.add_argument('--device', default='', help='cuda device, i.e. 0 or 0,1,2,3 or cpu')
parser.add_argument('--view-img', action='store_true', help='display results')
parser.add_argument('--save-txt', action='store_true', help='save results to *.txt')
parser.add_argument('--save-conf', action='store_true', help='save confidences in --save-txt labels')
parser.add_argument('--save-crop', action='store_true', help='save cropped prediction boxes')
parser.add_argument('--nosave', action='store_true', help='do not save images/videos')
parser.add_argument('--classes', nargs='+', type=int, help='filter by class: --class 0, or --class 0 2 3')
parser.add_argument('--agnostic-nms', action='store_true', help='class-agnostic NMS')
parser.add_argument('--augment', action='store_true', help='augmented inference')
parser.add_argument('--update', action='store_true', help='update all models')
parser.add_argument('--project', default='runs/detect', help='save results to project/name')
parser.add_argument('--name', default='exp', help='save results to project/name')
parser.add_argument('--exist-ok', action='store_true', help='existing project/name ok, do not increment')
opt = parser.parse_args()
```
这里使用Python的argparse库来定义输入参数,包括模型权重文件、输入数据源、推理尺寸、置信度阈值、NMS阈值等。
3. 加载模型
```python
# 加载模型
model = attempt_load(opt.weights, map_location=device) # load FP32 model
imgsz = check_img_size(opt.img_size, s=model.stride.max()) # check img_size
if device.type != 'cpu':
model(torch.zeros(1, 3, imgsz, imgsz).to(device).type_as(next(model.parameters()))) # run once
```
这里使用`attempt_load()`函数来加载模型,该函数会根据传入的权重文件路径自动选择使用哪个版本的YoloV7模型。同时,这里还会检查输入图片的大小是否符合模型的要求。
4. 设置计算设备
```python
# 设置计算设备
device = select_device(opt.device)
half = device.type != 'cpu' # half precision only supported on CUDA
# Initialize model
model.to(device).eval()
```
这里使用`select_device()`函数来选择计算设备(GPU或CPU),并将模型移动到选择的设备上。
5. 加载数据集
```python
# 加载数据集
if os.path.isdir(opt.source):
dataset = LoadImages(opt.source, img_size=imgsz)
else:
dataset = LoadStreams(opt.source, img_size=imgsz)
```
根据输入参数中的数据源,使用`LoadImages()`或`LoadStreams()`函数来加载数据集。这两个函数分别支持从图片文件夹或摄像头/视频中读取数据。
6. 定义类别和颜色
```python
# 定义类别和颜色
names = model.module.names if hasattr(model, 'module') else model.names
colors = [[np.random.randint(0, 255) for _ in range(3)] for _ in names]
```
这里从模型中获取类别名称,同时为每个类别随机生成一个颜色,用于在图片中绘制框和标签。
7. 定义输出文件夹
```python
# 定义输出文件夹
save_dir = Path(increment_path(Path(opt.project) / opt.name, exist_ok=opt.exist_ok)) # increment run
(save_dir / 'labels' if opt.save_txt else save_dir).mkdir(parents=True, exist_ok=True) # make dir
```
这里使用`increment_path()`函数来生成输出文件夹的名称,同时创建相应的文件夹。
8. 开始推理
```python
# 开始推理
for path, img, im0s, vid_cap in dataset:
t1 = time_synchronized()
# 图像预处理
img = torch.from_numpy(img).to(device)
img = img.half() if half else img.float()
img /= 255.0
if img.ndimension() == 3:
img = img.unsqueeze(0)
# 推理
pred = model(img)[0]
# 后处理
pred = non_max_suppression(pred, opt.conf_thres, opt.iou_thres, classes=opt.classes, agnostic=opt.agnostic_nms)
t2 = time_synchronized()
# 处理结果
for i, det in enumerate(pred): # detections per image
if webcam: # batch_size >= 1
p, s, im0 = path[i], f'{i}: ', im0s[i].copy()
else:
p, s, im0 = path, '', im0s
save_path = str(save_dir / p.name)
txt_path = str(save_dir / 'labels' / p.stem) + ('' if dataset.mode == 'image' else f'_{counter}') + '.txt'
if det is not None and len(det):
det[:, :4] = scale_coords(img.shape[2:], det[:, :4], im0.shape).round()
for *xyxy, conf, cls in reversed(det):
c = int(cls)
label = f'{names[c]} {conf:.2f}'
plot_one_box(xyxy, im0, label=label, color=colors[c], line_thickness=3)
if opt.save_conf:
with open(txt_path, 'a') as f:
f.write(f'{names[c]} {conf:.2f}\n')
if opt.save_crop:
w = int(xyxy[2] - xyxy[0])
h = int(xyxy[3] - xyxy[1])
x1 = int(xyxy[0])
y1 = int(xyxy[1])
x2 = int(xyxy[2])
y2 = int(xyxy[3])
crop_img = im0[y1:y2, x1:x2]
crop_path = save_path + f'_{i}_{c}.jpg'
cv2.imwrite(crop_path, crop_img)
# 保存结果
if opt.nosave:
pass
elif dataset.mode == 'images':
cv2.imwrite(save_path, im0)
else:
if vid_path != save_path: # new video
vid_path = save_path
if isinstance(vid_writer, cv2.VideoWriter):
vid_writer.release() # release previous video writer
fourcc = 'mp4v' # output video codec
fps = vid_cap.get(cv2.CAP_PROP_FPS)
w = int(vid_cap.get(cv2.CAP_PROP_FRAME_WIDTH))
h = int(vid_cap.get(cv2.CAP_PROP_FRAME_HEIGHT))
vid_writer = cv2.VideoWriter(save_path, cv2.VideoWriter_fourcc(*fourcc), fps, (w, h))
vid_writer.write(im0)
# 打印结果
print(f'{s}Done. ({t2 - t1:.3f}s)')
# 释放资源
if cv2.waitKey(1) == ord('q'): # q to quit
raise StopIteration
elif cv2.waitKey(1) == ord('p'): # p to pause
cv2.waitKey(-1)
```
这里使用一个循环来遍历数据集中的所有图像或视频帧,对每张图像或视频帧进行以下操作:
- 图像预处理:将图像转换为PyTorch张量,并进行归一化和类型转换。
- 推理:将图像张量传入模型进行推理,得到预测结果。
- 后处理:对预测结果进行非极大值抑制、类别筛选等后处理操作,得到最终的检测结果。
- 处理结果:对每个检测框进行标签和颜色的绘制,同时可以选择保存检测结果的图片或视频以及标签信息的TXT文件。
- 释放资源:根据按键输入决定是否退出或暂停程序。
9. 总结
以上就是YoloV7的测试脚本test.py的详细解释,通过这个脚本可以方便地测试已经训练好的模型,并对检测结果进行可视化和保存等操作。
相关推荐
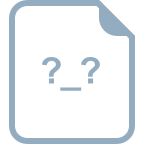
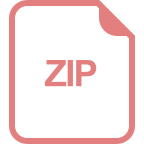












