const_cast<int&>(i)
时间: 2023-10-25 16:04:12 浏览: 47
const_cast<int>是C++中的一种类型转换操作符,用于去除变量的常量性。在C++中,const修饰符可以用于定义常量对象,即该对象的值在定义后不能再被修改。但有时需要修改一个被const修饰的对象的值,这时就可以使用const_cast<int>。
const_cast<int>可以用于转换常量指针或引用,以便能够对其所指对象进行修改。例如,假设有一个const int类型的指针或引用,如果希望修改所指对象的值,可以使用const_cast<int>进行类型转换,将其转换为int类型的指针或引用。
使用const_cast<int>需要非常谨慎,因为它会绕过const修饰符的限制,可能导致程序运行时出现未定义的行为。当使用const_cast<int>转换一个常量对象时,如果试图修改该对象的值,可能会导致程序崩溃或产生不可预料的结果。
总之,const_cast<int>是C++中一种用于去除常量性的类型转换操作符,可以将const修饰的指针或引用转换为非常量的指针或引用,以便能够修改所指对象的值。使用时需要慎重,确保转换后的对象可以被安全地修改。
相关问题
static_cast<int>(x)
`static_cast<int>(x)` 是 C++ 中的一种类型转换操作符,用于将变量 `x` 转换为整数类型 `int`。
这个类型转换操作符可以用于以下情况:
1. 当你需要将一个浮点数或其他数值类型转换为整数类型时,可以使用 `static_cast<int>(x)`。
2. 当你需要将一个指针类型转换为整数类型时,也可以使用 `static_cast<int>(x)`。这种情况下,指针的值将被转换为整数值,但请注意,这可能会导致精度丢失或未定义行为。
3. 当你需要将一个枚举类型转换为整数类型时,同样可以使用 `static_cast<int>(x)`。
需要注意的是,`static_cast` 并不会执行运行时检查,因此在进行类型转换时要确保转换是安全和合理的,否则可能会导致错误或未定义行为。如果需要进行更复杂的类型转换,可以考虑使用其他类型转换操作符,如 `dynamic_cast`、`reinterpret_cast` 或 `const_cast`。
针对下面这段代码,怎么优化map表和funAll函数const string FUNC1 = "func1"; const string FUNC2 = "func2"; const string FUNC3 = "func3"; int func1(int a, int b) { return a + b; } float func2(int a, float b, float c) { return float(a) + b + c; } int func3(int a, const char *b) { int result = a; auto length = strlen(b); for (size_t i = 0; i < length; i++) { result += b[i] - '0'; } return result; } map<string, function<any(vector<any>)>> funcMap = { {FUNC1, [](vector<any> args) { return func1(any_cast<int>(args[0]), any_cast<int>(args[1])); }}, {FUNC2, [](vector<any> args) { return func2(any_cast<int>(args[0]), any_cast<float>(args[1]), any_cast<float>(args[2])); }}, {FUNC3, [](vector<any> args) { return func3(any_cast<int>(args[0]), any_cast<char *>(args[1])); }} }; int funAll(const string &key, const vector<any> args) { if (funcMap.find(key) == funcMap.end()) { return -1; } auto func = funcMap[key]; auto result = func(args); cout << any_cast<float>(result) << endl; return 0; }
函数名, vector<any> args)
{
auto func = funcMap.find(funcName);
if (func != funcMap.end())
{
return any_cast<int>((func->second)(args));
}
return 0;
}
针对这个问题,可以进行以下优化:
1.修改map表:可以使用unordered_map代替map,因为unordered_map使用哈希表,可以快速查找元素,而map使用红黑树,查找元素比较慢。
2.修改函数定义:可以将函数返回值类型设置为auto,让编译器自动推导返回值类型。
3.修改funAll函数:可以使用auto关键字来自动推导func的类型,以及使用const引用参数来避免不必要的复制。同时可以将if语句中的find()和end()合并为一个语句,提高代码的可读性和效率。
优化后的代码如下:
const string FUNC1 = "func1";
const string FUNC2 = "func2";
const string FUNC3 = "func3";
auto func1(int a, int b) {
return a + b;
}
auto func2(int a, float b, float c) {
return float(a) + b + c;
}
auto func3(int a, const char* b) {
int result = a;
auto length = strlen(b);
for (size_t i = 0; i < length; i++) {
result += b[i] - '0';
}
return result;
}
unordered_map<string, function<any(vector<any>&)>> funcMap = {
{FUNC1, [](vector<any>& args) { return func1(any_cast<int>(args[0]), any_cast<int>(args[1])); }},
{FUNC2, [](vector<any>& args) { return func2(any_cast<int>(args[0]), any_cast<float>(args[1]), any_cast<float>(args[2])); }},
{FUNC3, [](vector<any>& args) { return func3(any_cast<int>(args[0]), any_cast<const char*>(args[1])); }}
};
int funAll(const string& funcName, vector<any>& args) {
auto func = funcMap.find(funcName);
if (func != funcMap.end()) {
return any_cast<int>((func->second)(args));
}
return 0;
}
相关推荐
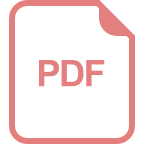
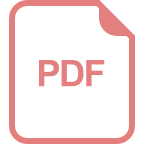
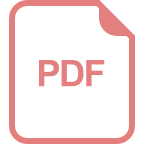
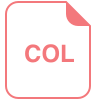












