qgraphicsitem旋转
时间: 2024-04-10 11:12:45 浏览: 39
QGraphicsItem可以通过旋转来改变其在场景中的方向。可以通过使用QTransform类中的rotate()函数来实现旋转效果。旋转中心可以使用boundingRect().center()来获取,并使用setTransformOriginPoint()来设置旋转中心。旋转角度可以使用setRotation()来设置。具体步骤如下:
1. 确定旋转中心的坐标,可以使用boundingRect().center()来获取[3]。
2. 在mousePressEvent(QMouseEvent *e)中获取按下时的坐标,即pressPos。
3. 在mouseMoveEvent(QMouseEvent *e)中获取当前坐标,即movePos。
4. 使用centerPos、pressPos和movePos三点来计算旋转角度,即rotateAngle。
5. 创建一个QTransform对象,并使用rotate()函数将其沿着Z轴旋转指定的角度rotateAngle。然后使用setTransform()将其应用到QGraphicsItem上。
6. 完成旋转。
通过以上步骤可以实现对QGraphicsItem的旋转效果。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
相关问题
QGraphicsItem 镜像旋转
在 Qt 中,QGraphicsItem 类提供了对图形项进行操作的接口,也可以通过 QTransform 对象对 QGraphicsItem 进行镜像旋转。具体步骤如下:
1. 创建一个 QGraphicsItem 对象;
2. 通过 QGraphicsScene 将 QGraphicsItem 加入到场景中;
3. 在 QGraphicsItem 的 paint() 函数中,使用 QPainter 绘制需要进行镜像旋转的图像;
4. 在 QGraphicsItem 的 paint() 函数中,创建一个 QTransform 对象,并使用 rotate() 和 scale() 函数进行镜像旋转操作,例如:
```cpp
QTransform transform;
transform.rotate(180, Qt::XAxis);
transform.scale(-1, 1);
painter->setTransform(transform);
```
其中,rotate() 函数表示绕 X 轴旋转 180 度,scale() 函数表示沿 X 轴进行水平翻转。
5. 将镜像旋转后的图像绘制到 QGraphicsItem 上,例如:
```cpp
painter->drawPixmap(QPointF(0, 0), pixmap);
```
6. 最后将 QGraphicsItem 添加到 QGraphicsScene 中,例如:
```cpp
scene->addItem(item);
```
注意:在进行镜像旋转操作时,需要将 QPainter 对象的坐标原点移动到图像的中心点,例如:
```cpp
painter->translate(boundingRect().center());
```
这样可以确保图像在进行旋转和翻转操作后仍然能够正常显示。
如何旋转QGraphicsItem
要旋转QGraphicsItem,可以使用QGraphicsItem的setRotation()函数。该函数接受一个浮点数参数,表示以顺时针方向旋转的角度。例如,如果要将一个QGraphicsItem旋转90度,可以这样写:
```python
item.setRotation(90)
```
如果要旋转QGraphicsItem的中心点,可以使用setTransformOriginPoint()函数设置旋转中心。例如,如果要将旋转中心设置为QGraphicsItem的中心点,可以这样写:
```python
item.setTransformOriginPoint(item.boundingRect().center())
```
相关推荐
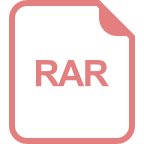












