对称加密python代码实现
时间: 2024-10-23 22:05:53 浏览: 21
对称加密是一种常用的加密技术,其中使用相同的密钥(secret key)进行加密和解密数据。Python中有多种库可以用于对称加密,如`cryptography`库。下面是一个简单的AES(Advanced Encryption Standard)对称加密的示例:
```python
from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes
from cryptography.hazmat.backends import default_backend
# 加密函数
def encrypt(plaintext, key):
backend = default_backend()
cipher_suite = Cipher(algorithms.AES(key), modes.ECB(), backend=backend)
encryptor = cipher_suite.encryptor() # 创建加密器
ciphertext = encryptor.update(plaintext) + encryptor.finalize() # 加密数据
return ciphertext
# 解密函数
def decrypt(ciphertext, key):
backend = default_backend()
cipher_suite = Cipher(algorithms.AES(key), modes.ECB(), backend=backend)
decryptor = cipher_suite.decryptor() # 创建解密器
plaintext = decryptor.update(ciphertext) + decryptor.finalize() # 解密数据
return plaintext.decode('utf-8') # 将字节转换回字符串(假设输入的是UTF-8编码)
# 示例
key = b'sixteen_byte_key' # 16字节的AES密钥
plaintext = "This is a secret message"
ciphertext = encrypt(plaintext.encode('utf-8'), key)
print("Encrypted:", ciphertext)
decrypted_text = decrypt(ciphertext, key)
print("Decrypted:", decrypted_text)
阅读全文
相关推荐
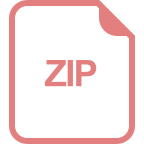
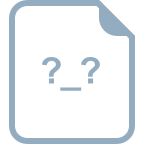

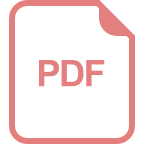
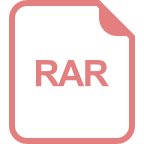
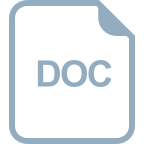











