用keil编写stm32串口接收以及发送代码
时间: 2023-12-04 08:05:12 浏览: 47
以下是一个简单的示例代码,用于在Keil中编写STM32串口接收和发送:
```c
#include "stm32fxxx.h"
#include "stm32fxxx_usart.h"
// 定义串口号
#define USARTx USART2
// 定义发送和接收缓冲区大小
#define BUFFER_SIZE 100
// 定义发送和接收缓冲区
uint8_t tx_buffer[BUFFER_SIZE];
uint8_t rx_buffer[BUFFER_SIZE];
// 串口发送函数
void USART_SendString(const uint8_t* str) {
while (*str) {
// 等待数据寄存器为空
while (!(USARTx->SR & USART_SR_TXE));
// 发送数据
USART_SendData(USARTx, *str++);
}
}
// 串口接收函数
void USART_ReceiveString(uint8_t* str) {
uint8_t i = 0;
while (1) {
// 等待接收到数据
while (!(USARTx->SR & USART_SR_RXNE));
// 读取接收到的数据
str[i] = USART_ReceiveData(USARTx);
// 判断是否接收到换行符
if (str[i] == '\n') {
str[i] = '\0'; // 字符串结束符
break;
}
i++;
}
}
int main(void) {
// 初始化串口引脚和时钟等配置
// 配置波特率、数据位、停止位等参数
USART_InitTypeDef USART_InitStructure;
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USARTx, &USART_InitStructure);
// 使能串口
USART_Cmd(USARTx, ENABLE);
while (1) {
// 接收数据
USART_ReceiveString(rx_buffer);
// 处理接收到的数据
// ...
// 发送数据
USART_SendString(tx_buffer);
// 清空缓冲区
memset(rx_buffer, 0, sizeof(rx_buffer));
memset(tx_buffer, 0, sizeof(tx_buffer));
}
}
```
在这个示例代码中,我们定义了一个串口号`USARTx`,并定义了发送和接收缓冲区`tx_buffer`和`rx_buffer`。`USART_SendString()`函数用于发送字符串,`USART_ReceiveString()`函数用于接收字符串。在主函数中,我们通过调用这两个函数来实现串口通信。
你需要根据你的具体硬件和需求进行相应的配置和修改。请确保正确设置引脚、时钟和其他相关参数,以及适配你的具体芯片型号。
希望这个示例代码能帮助到你!如果有任何问题,请随时提问。
相关推荐
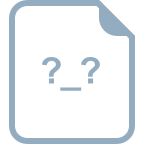
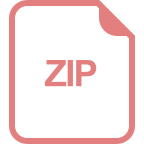
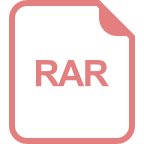
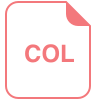
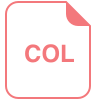
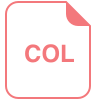
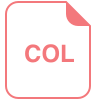
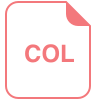









