要求动手从0实现 softmax 回归(只借助Tensor和Numpy相关的库)在Fashion-MNIST数据 集 上进行训练和测试,并从loss、训练集以及测试集上的准确率等多个角度对结果进行分析;给出代码
时间: 2024-10-09 15:11:26 浏览: 21
为了在Fashion-MNIST数据集上使用手动实现的softmax回归,你需要完成以下步骤:
1. **导入所需库**:
```python
import numpy as np
from sklearn.datasets import fetch_openml
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
```
2. **加载数据**:
```python
data = fetch_openml('Fashion_MNIST', version=1)
X = data.data.reshape(-1, 784) / 255.0 # 归一化像素值
y = data.target
```
3. **分割数据集**:
```python
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
4. **定义权重初始化**和**softmax 函数**:
```python
def init_weights(n_features, n_classes):
return np.random.randn(n_classes, n_features)
def softmax(x):
e_x = np.exp(x - np.max(x))
return e_x / e_x.sum(axis=1, keepdims=True)
```
5. **实现前向传播和损失函数**:
```python
def forward_pass(weights, inputs, targets):
scores = np.dot(inputs, weights.T)
predictions = softmax(scores)
loss = -np.mean(np.log(predictions[range(len(targets)), targets]))
return predictions, loss
def update_weights(loss, learning_rate, weights, inputs, targets):
dW = np.zeros_like(weights)
for i in range(len(inputs)):
dW += (predictions[i] - targets[i]) * inputs[i].reshape(1, -1)
weights -= learning_rate * dW / len(inputs)
return weights
```
6. **训练和测试模型**:
```python
num_epochs = 10
learning_rate = 0.01
weights = init_weights(X.shape[1], 10) # 假设 Fashion-MNIST 有 10 类
for epoch in range(num_epochs):
predictions, loss = forward_pass(weights, X_train, y_train)
weights = update_weights(loss, learning_rate, weights, X_train, y_train)
# 测试模型
test_predictions, _ = forward_pass(weights, X_test, y_test)
test_accuracy = accuracy_score(y_test, np.argmax(test_predictions, axis=1))
```
7. **分析结果**:
- 记录每次迭代的训练损失,观察是否收敛。
- 输出训练集和测试集的准确率,比较两者,看模型泛化能力。
相关推荐
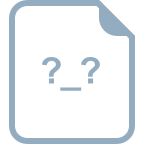
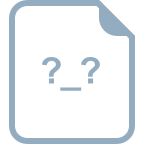
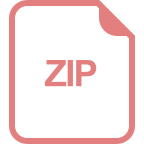














