用 C 语言编写程序,输入一个有无序的顺序表,将其中的重复数据只保留一个,算法时间复杂度为 O(n^2)或 O(n^3)皆可。
时间: 2024-09-28 09:07:37 浏览: 4
在C语言中,如果你想要删除一个有序列表(升序排列)中的重复元素并保持O(n^2)的时间复杂度,可以使用简单遍历的方法,比如两指针法。这里是一个基本的示例:
```c
#include <stdio.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
Node* removeDuplicates(Node* head) {
if (head == NULL || head->next == NULL) return head; // 如果链表为空或只有一个元素,直接返回
Node* current = head;
Node* runner = head->next;
while (runner != NULL) { // 遍历整个链表
if (current->data == runner->data) {
current->next = runner->next; // 将当前节点指向下一个非重复节点
free(runner); // 释放重复节点的内存
} else {
current = runner; // 更新current到当前处理的节点
}
runner = runner->next;
}
return head;
}
void printList(Node* node) {
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
printf("\n");
}
int main() {
Node* list = createYourList(); // 假设createYourList函数已创建了一个有重复值的链表
removeDuplicates(list);
printList(list);
return 0;
}
```
对于无序列表,由于无法保证元素顺序,我们通常需要先对列表排序,这时时间复杂度会变为O(n log n),然后按照上面的步骤去除重复。如果允许O(n^3)的时间复杂度,可以考虑使用哈希集合(如数组或关联数组)辅助查找。
相关推荐
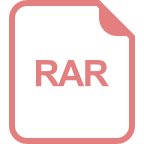
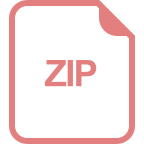
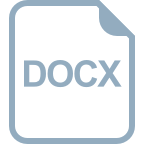



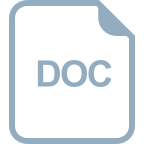
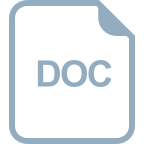
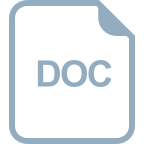
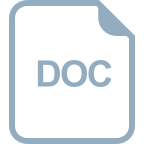
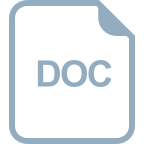
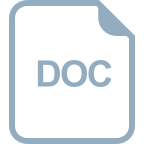
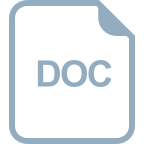
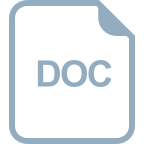
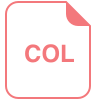
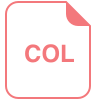
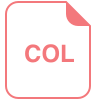
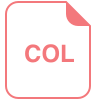