java根据文件路径将多个文件合并为一个pdf文件的方法
时间: 2024-04-10 12:33:44 浏览: 27
要将多个文件合并为一个 PDF 文件,可以使用第三方库 iTextPDF。以下是一个示例代码:
首先,确保你已将 iTextPDF 添加到你的项目中。你可以通过 Maven 或手动下载 JAR 文件的方式添加依赖。
```java
import com.itextpdf.text.Document;
import com.itextpdf.text.DocumentException;
import com.itextpdf.text.pdf.PdfCopy;
import com.itextpdf.text.pdf.PdfReader;
import java.io.FileOutputStream;
import java.io.IOException;
public class PDFMerger {
public static void main(String[] args) {
String[] sourceFiles = {"path/to/file1.pdf", "path/to/file2.pdf", "path/to/file3.pdf"};
String targetFile = "path/to/merged.pdf";
mergePDFs(sourceFiles, targetFile);
System.out.println("Files merged successfully.");
}
public static void mergePDFs(String[] sourceFiles, String targetFile) {
Document document = new Document();
try {
PdfCopy copy = new PdfCopy(document, new FileOutputStream(targetFile));
document.open();
for (String sourceFile : sourceFiles) {
PdfReader reader = new PdfReader(sourceFile);
copy.addDocument(reader);
reader.close();
}
copy.close();
document.close();
} catch (IOException | DocumentException e) {
e.printStackTrace();
}
}
}
```
在上面的示例中,我们首先指定了要合并的源文件的路径数组 `sourceFiles`,以及目标文件的路径 `targetFile`。然后,我们定义了一个 `mergePDFs` 方法来执行合并操作。
在 `mergePDFs` 方法中,我们首先创建一个 `Document` 对象,并使用 `PdfCopy` 对象将其与目标文件关联起来。然后,我们遍历源文件数组,对于每个源文件,我们创建一个 `PdfReader` 对象,并使用 `copy.addDocument` 方法将其添加到目标文件中。最后,我们关闭 `PdfCopy` 和 `Document` 对象。
请确保替换示例代码中的文件路径为你实际的文件路径,并根据你的项目配置进行必要的导入和依赖管理。
相关推荐
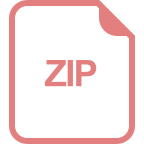
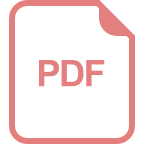
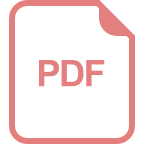














