int赋值std::string
时间: 2024-08-12 08:04:28 浏览: 97
在C++中,`int`是一个基本数据类型,代表整数,而`std::string`是一个标准库中的对象,用于存储文本字符串。由于它们的数据类型不同,直接将`int`赋值给`std::string`通常是不允许的,因为它们不具备直接的转换规则。
如果你想将一个整数值转换成字符串并保存到`std::string`中,你需要使用`std::to_string()`函数。例如:
```cpp
int value = 42;
std::string strValue = std::to_string(value);
```
在这个例子中,`value`变量被转换成了字符串,并存储到了`strValue`中。如果你试图直接赋值,如`std::string myString = int;`,编译器会报错,因为这是类型的不匹配。
相关问题
写一个std::map<std::string, std::variant<int, std::string>>赋值并读取的demo
当使用`std::map`结合`std::variant`在C++中存储键值对时,你可以创建一个映射,其中键是`std::string`类型,值是一个可以是`int`或`std::string`类型的`std::variant`。以下是一个简单的示例,展示如何创建、添加、访问和读取这些数据:
```cpp
#include <iostream>
#include <map>
#include <variant>
#include <string>
using Value = std::variant<int, std::string>;
// 创建一个std::map实例
std::map<std::string, Value> myMap;
// 添加元素
void addElement(const std::string& key, int intValue) {
myMap[key] = intValue;
std::cout << "Added " << key << " with int value: " << intValue << std::endl;
}
void addElement(const std::string& key, const std::string& stringValue) {
myMap[key] = stringValue;
std::cout << "Added " << key << " with string value: " << stringValue << std::endl;
}
// 读取元素(假设值是int)
int getValueAsInt(const std::string& key) {
auto it = myMap.find(key);
if (it != myMap.end()) {
return std::get<int>(*it->second);
}
throw std::runtime_error("Key not found in the map");
}
// 读取元素(假设值是std::string)
std::string getValueAsString(const std::string& key) {
auto it = myMap.find(key);
if (it != myMap.end()) {
return std::get<std::string>(*it->second);
}
throw std::runtime_error("Key not found in the map");
}
int main() {
// 示例用法
addElement("key1", 100);
addElement("key2", "Hello");
// 读取并输出int值
try {
std::cout << "Value of key1 as int: " << getValueAsInt("key1") << std::endl;
} catch (const std::exception& e) {
std::cerr << e.what() << std::endl;
}
// 读取并输出string值
try {
std::cout << "Value of key2 as string: " << getValueAsString("key2") << std::endl;
} catch (const std::exception& e) {
std::cerr << e.what() << std::endl;
}
return 0;
}
```
在这个例子中,`addElement`函数用于向map中添加元素,`getValueAsInt`和`getValueAsString`分别用于根据键获取对应的整数或字符串值。注意,由于`std::variant`的值可能包含不同类型的值,因此在读取时需要指定类型进行解包。
c++ 给std::map<int,std::string>赋值
可以使用 insert 函数或者 [] 运算符来给 std::map<int,std::string> 赋值。具体实现如下:
```c++
#include <iostream>
#include <map>
#include <string>
int main() {
std::map<int, std::string> myMap;
// 使用 insert 函数赋值
myMap.insert(std::make_pair(1, "hello"));
myMap.insert(std::make_pair(2, "world"));
// 使用 [] 运算符赋值
myMap[3] = "C++";
// 遍历 map
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << it->first << ": " << it->second << std::endl;
}
return 0;
}
```
输出结果为:
```
1: hello
2: world
3: C++
```
阅读全文
相关推荐
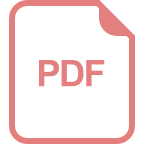
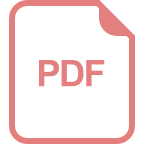
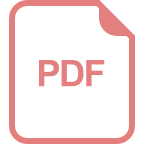













