如何用编程语言来模拟这种螺旋运动并计算位置和速度?
时间: 2024-09-06 18:02:37 浏览: 81
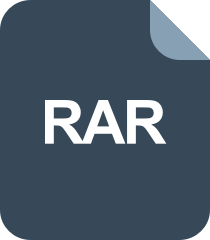
螺旋线运动轨迹控制梯形图程序
用编程语言模拟这种螺旋运动通常涉及以下步骤:
1. **初始化变量**:
- 时间 `time` 或者步长 `dt`
- 龙头位置 `head_position`
- 螺旋线参数,如螺距 `pitch`, 盘绕圈数 `loops` 及起点 `start_circle`
- 手把列表及其初始位置 `handles_positions`,包括龙头把手和其他把手
2. **函数定义**:
- `calculate_distance_to_head(hand_number)`:计算某个把手到龙头的距离
- `update_speed(hand_number)`:基于把手位置和螺线参数计算当前速度
- `update_position(hand_number)`:根据速度和时间更新把手位置
3. **循环计算**:
- 对于每个时间点(例如,每秒或每隔一步):
- 更新龙头位置
- 计算把手的位置(考虑螺旋线的圆周性和距离衰减)
- 存储所有把手的位置和速度到数据结构(如字典或数组)
4. **数据存储**:
- 使用适当的数据结构(如 pandas DataFrame)将数据组织起来,便于写入 Excel 文件。确保添加时间戳列以及六位小数精度。
5. **定时输出**:
- 每隔 0s, 60s, ..., 300s 输出指定位置和速度的数据到表格中。
这里可以使用 Python 编程语言,结合 numpy 或 pandas 库来简化数值计算和数据处理。以下是一个简单的伪代码示例:
```python
import time
import numpy as np
def spiral_movement(head_position, pitch, loops, handles_positions):
dt = 1 # 时间间隔
for t in range(int(300 / dt)):
head_position += dt
for hand_number, handle_position in enumerate(handles_positions):
distance_to_head = calculate_distance_to_head(hand_number, head_position)
speed = update_speed(distance_to_head, pitch)
new_position = update_position(handle_position, speed, dt)
handles_positions[hand_number] = new_position
# 如果需要,每60秒记录一次数据
if (t+1) % 60 == 0 or t+1 == 300:
record_data(t, handles_positions, hand_number)
# 具体的计算函数在这里
# ...
def record_data(time, positions, specific_hands):
# 创建DataFrame,存储数据到result1.xlsx
df = pd.DataFrame({'time': [time], 'positions': positions, 'specific_hands': specific_hands})
df.to_excel('result1.xlsx', index=False)
# 初始化数据和开始运动
...
```
这只是一个简化的例子,实际代码可能需要根据你的需求和数据结构进行调整。如果你需要详细的代码实现或者遇到具体问题,请随时提问。
阅读全文
相关推荐
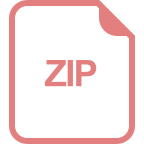
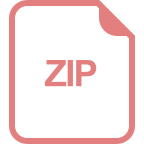
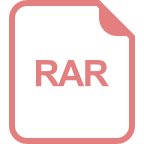
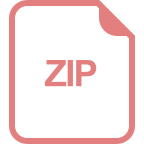
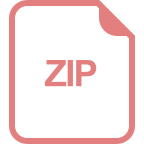
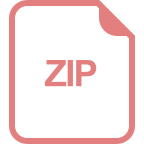
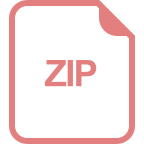
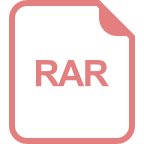
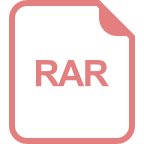
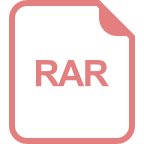
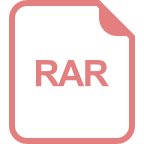
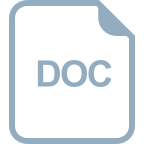
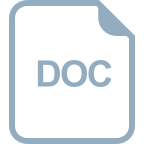
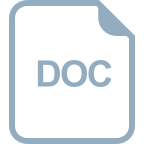
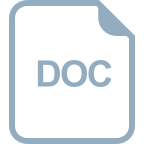
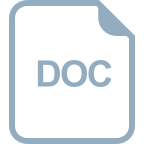
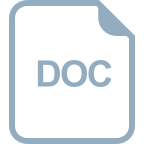
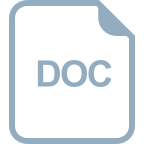