PTA中7-1 jmu-ds-栈与队列-stack、queue与string小综合C语言代码
时间: 2024-10-12 20:08:59 浏览: 4
在程序设计(Programming Technology Assessment, PTA)中,题目通常涉及对基本数据结构如栈(stack)和队列(queue)的理解以及它们在C语言中的实现。这两个数据结构在处理序列元素时非常有用,尤其是当需要按照特定顺序添加和删除元素时。
对于7-1题目的描述不够明确,但我可以给你一个简单的C语言代码示例,展示如何用数组模拟栈和队列,并附上一个字符串操作的小例子:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 10
// 定义栈
typedef struct {
char* items;
int top;
int size;
} Stack;
Stack* create_stack() {
Stack* s = (Stack*)malloc(sizeof(Stack));
s->items = (char*)malloc(MAX_SIZE * sizeof(char));
s->top = -1;
s->size = 0;
return s;
}
// 入栈操作
void push(Stack* s, char item) {
if (s->top == MAX_SIZE - 1) {
printf("Stack overflow.\n");
return;
}
s->items[++s->top] = item;
s->size++;
}
// 出栈操作
char pop(Stack* s) {
if (s->top == -1) {
printf("Stack underflow.\n");
return '\0';
}
char item = s->items[s->top];
s->items[s->top] = '\0'; // 清空字符以便下次入栈
s->top--;
return item;
}
// 队列
typedef struct {
char* front;
char* rear;
int count;
} Queue;
Queue* create_queue() {
Queue* q = (Queue*)malloc(sizeof(Queue));
q->front = q->rear = (char*)malloc(MAX_SIZE * sizeof(char));
q->count = 0;
return q;
}
// 入队
void enqueue(Queue* q, char item) {
if (q->rear == q->front + MAX_SIZE - 1) {
printf("Queue is full.\n");
return;
}
*(q->rear++) = item; // 增加指针并保存元素
q->count++;
}
// 出队
char dequeue(Queue* q) {
if (q->count == 0) {
printf("Queue is empty.\n");
return '\0';
}
char item = *(q->front++);
q->count--;
return item;
}
int main() {
Stack* stack = create_stack();
char str[] = "Hello";
for (int i = 0; i < strlen(str); i++) {
push(stack, str[i]);
printf("Pushed '%c'\n", str[i]);
}
Queue* queue = create_queue();
for (int i = strlen(str) - 1; i >= 0; i--) {
enqueue(queue, str[i]);
printf("Enqueued '%c'\n", str[i]);
}
while (!isempty(stack)) {
char popped = pop(stack);
printf("Popped from stack: '%c'\n", popped);
}
while (!isempty(queue)) {
char dequeued = dequeue(queue);
printf("Dequeued from queue: '%c'\n", dequeued);
}
free(stack);
free(queue);
return 0;
}
// 用于检查是否栈或队列为空的辅助函数
int isempty(struct _Stack* s) { return s->top == -1; }
int isempty(Queue* q) { return q->count == 0; }
相关推荐
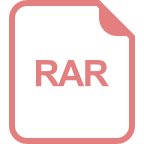
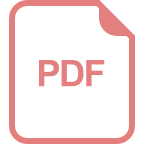














