分别用不动点迭代法、Newton 法、割线法求解函数f(x)=exp(x)-x-5的零点,编写MATLAB 程序,比较计算结果
时间: 2024-12-18 20:32:08 浏览: 13
不动点迭代法通常用于求解方程 \( g(x) = x - f(x) \) 的根,对于 \( f(x) = e^x - x - 5 \),我们可以设置 \( g(x) = x \)。以下是简单的 MATLAB 代码实现不动点迭代:
```matlab
function [root, iterations] = fixed_point_iterate(f, guess, tolerance, max_iters)
root = guess;
count = 0;
while abs(f(root)) > tolerance && count < max_iters
root = root - f(root);
count = count + 1;
end
iterations = count;
end
% 函数f
f = @(x) exp(x) - x - 5;
% 初始猜测值
guess = 1; % 或者其他合理猜测值,例如0或1.618
% 设置迭代参数
tolerance = 1e-6;
max_iters = 1000;
[zero_fixed, iters_fixed] = fixed_point_iterate(f, guess, tolerance, max_iters);
disp(['Fixed Point Iteration Result: ', num2str(zero_fixed), ' (', num2str(iters_fixed), ' iterations)']);
```
接下来是 Newton 法(牛顿-拉弗森法),它需要导数信息:
```matlab
function [newton_root] = newton_method(f, df, guess, tolerance, max_iters)
if nargin < 4 || nargin > 5
error('Invalid number of input arguments');
end
root = guess;
count = 0;
while abs(f(root)) > tolerance && count < max_iters
newton_root = root - f(root) / df(root);
root = newton_root;
count = count + 1;
end
if count == max_iters
disp(['Newton Method Converged after maximum iterations. Final result: ', num2str(newton_root)]);
else
disp(['Newton Method Result: ', num2str(newton_root), ' (', num2str(count), ' iterations)']);
end
end
% 函数f的导数
df = @(x) exp(x) - 1;
[zero_newton, iters_newton] = newton_method(f, df, guess, tolerance, max_iters);
```
最后是割线法(也称为二分法),适用于单调函数:
```matlab
function [zero_bisection, iters_bisection] = bisection_method(f, a, b, tolerance, max_iters)
if nargin < 4 || nargin > 5
error('Invalid number of input arguments');
end
if f(a) * f(b) >= 0
disp('Bisection method requires the function to change sign in the interval.');
return;
end
midpoint = (a + b) / 2;
root = midpoint;
count = 0;
while abs(f(root)) > tolerance && count < max_iters
if f(midpoint) * f(root) < 0
b = root;
else
a = root;
end
root = (a + b) / 2;
count = count + 1;
end
if count == max_iters
disp(['Bisection Method Converged after maximum iterations. Final result: ', num2str(root)]);
else
disp(['Bisection Method Result: ', num2str(root), ' (', num2str(count), ' iterations)']);
end
end
[zero_bisection, iters_bisection] = bisection_method(@(x) f(x), guess, guess*1.1, tolerance, max_iters);
```
现在你可以运行这三段代码,并比较它们的结果。每种方法可能会因为初始猜测、函数特性以及算法收敛速度而得出不同的数值和迭代次数。
阅读全文
相关推荐
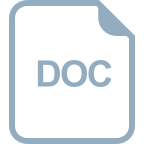
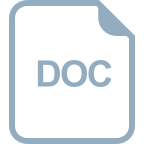
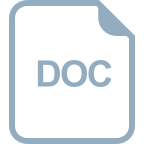
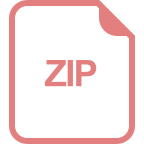
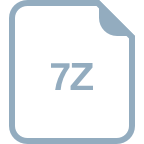
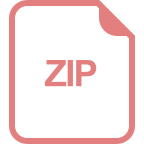
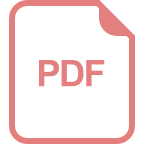
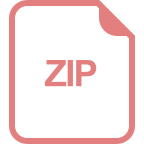
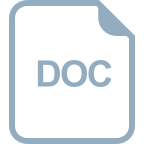
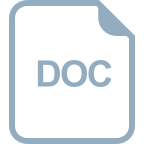
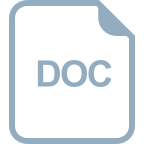
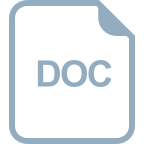
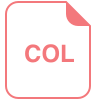
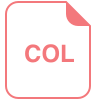
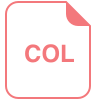
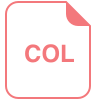


