给我讲一下回溯法01背包的代码
时间: 2024-12-25 12:23:52 浏览: 8
回溯法在解决0/1背包问题时是一种常见的动态规划解决方案。这个算法用于寻找所有可能的物品组合,使得它们的总价值不超过背包的最大容量,同时每种物品至多取一件。
以下是一个简单的C++回溯法实现01背包问题的例子:
```cpp
#include <iostream>
#include <vector>
// 定义背包的状态和值
struct Item {
int value; // 物品的价值
int weight; // 物品的重量
bool operator<(const Item &other) const {
return weight == other.weight ? value < other.value : weight < other.weight;
}
};
bool canFit(Item item, int capacity, std::vector<Item>& items) {
if (item.weight <= capacity) {
return true;
} else {
for (Item it : items) {
if (it.weight + item.weight <= capacity) {
return true;
}
}
return false;
}
}
void backtracking(int capacity, int index, std::vector<Item>& items, std::vector<bool>& taken, int totalValue) {
if (index == items.size()) { // 找到一种解或无法再添加
if (totalValue <= capacity) {
std::cout << "Solution found: Total value = " << totalValue << ", Capacity used = " << capacity << std::endl;
} else {
std::cout << "No solution found." << std::endl;
}
return;
}
// 取当前物品
taken[index] = true;
backtracking(capacity - items[index].weight, index + 1, items, taken, totalValue + items[index].value);
// 不取当前物品
taken[index] = false;
backtracking(capacity, index + 1, items, taken, totalValue);
}
int main() {
int capacity = 50; // 背包最大容量
std::vector<Item> items = {{60, 10}, {100, 20}, {120, 30}}; // 物品列表
std::vector<bool> taken(items.size(), false); // 存放是否选择物品的标记
// 排序物品以便于回溯
std::sort(items.begin(), items.end());
backtracking(capacity, 0, items, taken, 0); // 开始回溯
return 0;
}
```
在这个例子中,`backtracking` 函数采用了递归的方式,尝试每种可能的选择(取或不取当前物品),直到所有的物品都被考虑过或背包已满。当找到一种满足条件的组合时,就输出结果;否则表示无解。
阅读全文
相关推荐


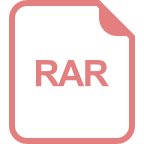

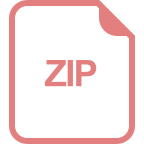
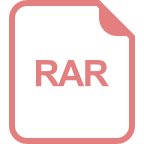










