python实现动态网页爬取实验 1.构建带参URL信息,组成UrI,爬取UrI对应网 页图片,并存入本地文件 2.读取不同text1和text2文件中的内容,获取不同Cookie1和Cookie2信息,爬取相关网页图片,并存入本地文件image1和image2
时间: 2024-11-03 14:23:46 浏览: 36
在Python中实现动态网页爬取通常会涉及到一些库,如requests、BeautifulSoup、selenium等。这里是一个简单的步骤概述:
1. **构建带参URL信息**:
使用`urllib.parse`模块处理URL参数。例如:
```python
from urllib.parse import urlencode
params = {'text1': 'value', 'text2': 'other_value'}
url_template = "http://example.com?{}".format(urlencode(params))
```
2. **爬取页面图片**:
- `requests.get(url, cookies=cookie1)`: 使用requests库发送GET请求,如果需要cookies, 将其作为参数传递。然后通过`response.content`获取图片数据。
- 存储图片到本地:
```python
with open('image1.jpg', 'wb') as f:
f.write(response.content)
```
3. **读取文本文件**:
使用内置的`open()`函数读取text1和text2文件的内容:
```python
with open('text1.txt', 'r') as file1, open('text2.txt', 'r') as file2:
cookie1 = file1.read()
cookie2 = file2.read()
```
4. **循环爬取并保存不同Cookie下的图片**:
对于每个cookie,按照上述步骤处理每一个URL,将图片分别保存为'image1.jpg' 和 'image2.jpg'。
```python
for i, cookie in enumerate([cookie1, cookie2]):
response = requests.get(url, cookies={'Cookie': cookie})
with open(f'image{i+1}.jpg', 'wb') as f:
f.write(response.content)
```
注意:动态网页往往有反爬虫机制,使用上述方法可能会遇到问题,比如需要处理验证码、登录验证等。在实际操作时,可能需要结合模拟浏览器的行为(如selenium配合ChromeDriver)来进行。
阅读全文
相关推荐
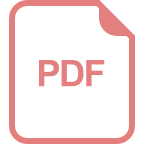
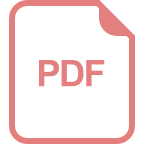
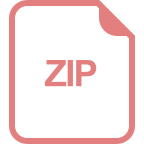
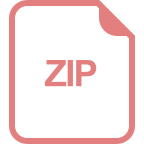
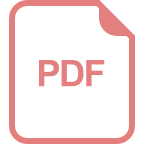
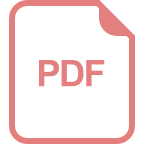
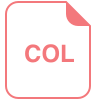
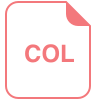
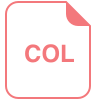
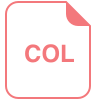
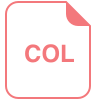
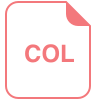
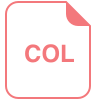
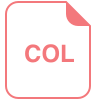




