C++分支界限算法解决旅行售货员问题代码
时间: 2024-11-29 21:36:26 浏览: 4
旅行售货员问题(Traveling Salesman Problem, TSP)是一个经典的组合优化问题,旨在找到一条最短路径,使得一个送货员能访问所有城市并返回起点一次。C++中的分支界限算法是一种通过搜索树结构来求解此类问题的方法,它结合了剪枝策略来减少搜索空间。
下面是一个简单的C++代码片段,展示了如何使用分支界限算法来解决TSP问题的基本框架:
```cpp
#include <vector>
#include <limits>
struct Node {
std::vector<int> path;
int cost;
bool operator<(const Node& other) const { return cost > other.cost; }
};
std::vector<int> tsp_brute_force(std::vector<int>& cities) {
// Brute force solution for small inputs
if (cities.size() <= 4) {
// Calculate all permutations and return the shortest one
return brute_force_permutations(cities);
}
// Branch and bound approach
Node best_node = { {}, cities.size() * cities[0], true };
std::vector<Node> frontier;
frontier.push_back({ cities });
while (!frontier.empty()) {
Node current = frontier.back();
frontier.pop_back();
// If we found a shorter path, update the best solution
if (current.cost < best_node.cost) {
best_node = current;
}
// Generate children by adding an edge to the next city not in the path
for (int i = 0; i < cities.size(); ++i) {
if (std::find(current.path.begin(), current.path.end(), i) == current.path.end()) {
Node child = current;
child.path.push_back(i);
child.cost = current.cost + distance(cities[current.path.back()], cities[i]);
frontier.push_back(child);
}
}
// Prune branches with higher costs
for (Node& node : frontier) {
if (node.cost > best_node.cost + std::numeric_limits<int>::max() / 2) {
break; // Early pruning
}
}
}
return best_node.path;
}
std::vector<int> brute_force_permutations(std::vector<int>& cities) {
// Implement a full permutation algorithm here
}
// A simple Euclidean distance function as an example
int distance(int x1, int y1, int x2, int y2) {
return std::sqrt((x1 - x2) * (x1 - x2) + (y1 - y2) * (y1 - y2));
}
```
注意:这个代码只是一个简化版的例子,实际应用中可能需要引入更复杂的剪枝规则、优先队列等数据结构,并可能需要处理更大规模的问题,这通常会借助于启发式算法如2-opt或Kruskal's algorithm来辅助决策。
阅读全文
相关推荐
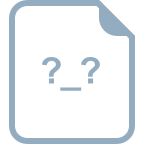
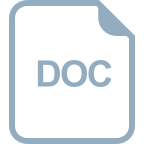
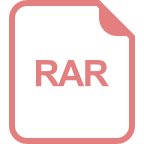


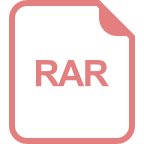
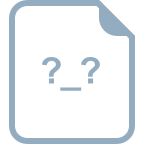

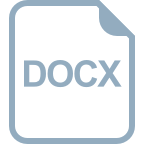
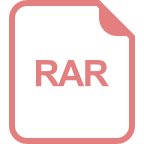
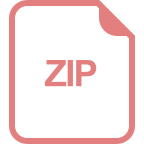
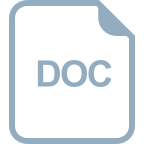

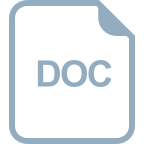
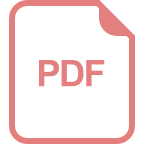
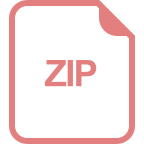
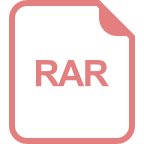
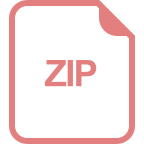
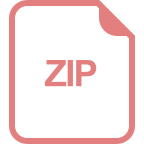