import numpy as np def replacezeroes(data): min_nonzero = np.min(data[np.nonzero(data)]) data[data == 0] = min_nonzero return data # Change the line below, based on U file # Foundation users set it to 20, ESI users set it to 21 LINE = 20 def read_scalar(filename): # Read file file = open(filename, 'r') lines_1 = file.readlines() file.close() num_cells_internal = int(lines_1[LINE].strip('\n')) lines_1 = lines_1[LINE + 2:LINE + 2 + num_cells_internal] for i in range(len(lines_1)): lines_1[i] = lines_1[i].strip('\n') field = np.asarray(lines_1).astype('double').reshape(num_cells_internal, 1) field = replacezeroes(field) return field def read_vector(filename): # Only x,y components file = open(filename, 'r') lines_1 = file.readlines() file.close() num_cells_internal = int(lines_1[LINE].strip('\n')) lines_1 = lines_1[LINE + 2:LINE + 2 + num_cells_internal] for i in range(len(lines_1)): lines_1[i] = lines_1[i].strip('\n') lines_1[i] = lines_1[i].strip('(') lines_1[i] = lines_1[i].strip(')') lines_1[i] = lines_1[i].split() field = np.asarray(lines_1).astype('double')[:, :2] return field if __name__ == '__main__': print('Velocity reader file') heights = [2.0, 1.5, 0.5, 0.75, 1.75, 1.25] total_dataset = [] # Read Cases for i, h in enumerate(heights, start=1): U = read_vector(f'U_{i}') nut = read_scalar(f'nut_{i}') cx = read_scalar(f'cx_{i}') cy = read_scalar(f'cy_{i}') h = np.ones(shape=(np.shape(U)[0], 1), dtype='double') * h temp_dataset = np.concatenate((U, cx, cy, h, nut), axis=-1) total_dataset.append(temp_dataset) total_dataset = np.reshape(total_dataset, (-1, 6)) print(total_dataset.shape) # Save data np.save('Total_dataset.npy', total_dataset) # Save the statistics of the data means = np.mean(total_dataset, axis=0).reshape(1, np.shape(total_dataset)[1]) stds = np.std(total_dataset, axis=0).reshape(1, np.shape(total_dataset)[1]) # Concatenate op_data = np.concatenate((means, stds), axis=0) np.savetxt('means', op_data, delimiter=' ') # Need to write out in OpenFOAM rectangular matrix format print('Means:') print(means) print('Stds:') print(stds)解析python代码,说明读取的数据文件格式
时间: 2024-04-17 21:29:34 浏览: 19
这段代码是一个用于读取数据文件的 Python 脚本。它包含了两个函数:`read_scalar(filename)` 和 `read_vector(filename)`,并使用了 `replacezeroes(data)` 函数来替换数组中的零值。脚本读取名为 `U_1`, `U_2`, ..., `U_6` 的文件,并根据文件中的数据生成一个包含各个高度的数据集。
函数 `read_scalar(filename)` 用于读取标量数据文件。它打开给定的文件,读取指定行数的数据,并将其存储在一个 NumPy 数组中。函数 `read_vector(filename)` 用于读取包含 x 和 y 分量的矢量数据文件。它也打开给定的文件,读取指定行数的数据,并将其存储在一个 NumPy 数组中。
在脚本的主程序中,一共读取了 6 个名为 `U`, `nut`, `cx`, `cy`, `h` 的文件,并将它们的数据分别存储在 `total_dataset` 列表中。然后,通过使用 NumPy 的函数将列表中的数据合并成一个包含 6 列的数组。最后,将合并后的数据保存为 `Total_dataset.npy` 文件,并计算并保存数据的均值和标准差。
这段代码假设数据文件的格式为文本文件,每行包含一个数据值。
相关问题
1、 凝聚层次聚类算法实现(非聚类库函数调用),要求算法输入:随机生成聚类的>=20个对象;算法输出:分类结果,聚类过程中得到的最短距离值以及距离矩阵。考虑三种不同距离计算方法进行聚类。
以下是非聚类库函数调用的凝聚层次聚类算法的Python实现。实现了三种不同距离计算方法:欧几里得距离、曼哈顿距离和切比雪夫距离。
```python
import numpy as np
import matplotlib.pyplot as plt
# 生成随机数据
np.random.seed(42)
data = np.random.rand(20, 2)
# 计算距离矩阵
def distance_matrix(data, metric='euclidean'):
n = data.shape[0]
distance_mat = np.zeros((n, n))
for i in range(n):
for j in range(i+1, n):
if metric == 'euclidean':
distance_mat[i, j] = np.linalg.norm(data[i, :] - data[j, :])
elif metric == 'manhattan':
distance_mat[i, j] = np.sum(np.abs(data[i, :] - data[j, :]))
elif metric == 'chebyshev':
distance_mat[i, j] = np.max(np.abs(data[i, :] - data[j, :]))
else:
raise ValueError('Invalid metric')
distance_mat += distance_mat.T
return distance_mat
# 凝聚层次聚类算法
def agglomerative_clustering(data, method='single', metric='euclidean'):
n = data.shape[0]
labels = np.arange(n)
distance_mat = distance_matrix(data, metric=metric)
min_distance = np.min(distance_mat[np.nonzero(distance_mat)])
clusters = [i for i in range(n)]
history = [(i,) for i in range(n)]
while len(clusters) > 1:
i, j = np.unravel_index(np.argmin(distance_mat), distance_mat.shape)
if method == 'single':
new_distance = np.min(distance_mat[i, labels == labels[j]])
elif method == 'complete':
new_distance = np.max(distance_mat[i, labels == labels[j]])
elif method == 'average':
new_distance = np.mean(distance_mat[i, labels == labels[j]])
else:
raise ValueError('Invalid method')
history.append((clusters[i], clusters[j]))
clusters[i] = tuple(sorted((clusters[i], clusters[j])))
clusters.pop(j)
labels[labels == labels[j]] = labels[i]
distance_mat = np.delete(distance_mat, j, axis=0)
distance_mat = np.delete(distance_mat, j, axis=1)
distance_mat[i, :] = np.minimum(distance_mat[i, :], distance_mat[j, :])
distance_mat[:, i] = distance_mat[i, :]
distance_mat[i, i] = 0
min_distance = min(min_distance, new_distance)
return clusters[0], min_distance, history
# 聚类并可视化结果
methods = ['single', 'complete', 'average']
metrics = ['euclidean', 'manhattan', 'chebyshev']
fig, axs = plt.subplots(3, 3, figsize=(12, 12))
for i, metric in enumerate(metrics):
for j, method in enumerate(methods):
clusters, min_distance, history = agglomerative_clustering(data, method=method, metric=metric)
axs[i, j].scatter(data[:, 0], data[:, 1], c=clusters)
axs[i, j].set_title(f'{method} ({metric})')
axs[i, j].set_xticks([])
axs[i, j].set_yticks([])
axs[i, j].text(0.05, 0.9, f'min_distance: {min_distance:.3f}\nclusters: {len(set(clusters))}', transform=axs[i, j].transAxes, fontsize=12, verticalalignment='top')
plt.show()
```
在上面的代码中,我们首先生成了20个随机的2维数据点,然后分别使用欧几里得距离、曼哈顿距离和切比雪夫距离计算了距离矩阵,并使用了三种不同的凝聚层次聚类方法(单链接、全链接和平均链接)进行了聚类。最后,我们将聚类结果可视化,并输出了最短距离值和聚类数目。
需要注意的是,由于凝聚层次聚类算法的时间复杂度较高,对于更大的数据集,可能需要进行优化。
LiTS2017数据集的路径为C:\Users\Administrator\Desktop\LiTS2017。 LiTS2017数据集里面包含Training Batch 1和Training Batch 2两个文件夹。Training Batch 1文件夹里面有图像,图像的拓展名为.nii。Training Batch 2文件夹里面有标签,标签拓展名为.nii。 请将我的LiTS2017数据集的3D图像变成2D、RGB三通道、的png格式的图像。数据集的3D标签变成2D单通道的png格式标签。 并将其裁剪为单个肝脏区域。同时,移除标签中无肝脏的图片以及肝脏在图片中面积占比小于2%的切片,对数据集进行增强操作,如旋转、翻转、缩放等,以扩充数据集,提高模型的泛化能力。 保存路径为C:\Users\Administrator\Desktop\2D-LiTS2017,请帮我创建好对应文件夹,并且将转换好的数据保存到对应的文件夹。
好的,根据您的要求,我可以为您提供以下Python代码来实现您的需求:
```python
import os
import numpy as np
import nibabel as nib
from PIL import Image
from skimage import transform
# 定义数据集路径和保存路径
data_path = "C:/Users/Administrator/Desktop/LiTS2017"
save_path = "C:/Users/Administrator/Desktop/2D-LiTS2017"
# 创建保存路径文件夹
if not os.path.exists(save_path):
os.makedirs(save_path)
# 定义函数将3D图像转换为2D、RGB三通道的png格式图像
def save_image(image, save_folder, name):
image_array = np.array(image.dataobj)
image_array = np.rot90(image_array, 1, axes=(1, 0))
image_array = (image_array - np.min(image_array)) / (np.max(image_array) - np.min(image_array)) * 255
image_array = np.uint8(image_array)
for i in range(image_array.shape[2]):
img = Image.fromarray(image_array[:, :, i])
img = img.convert("RGB")
img.save("{}/{}_{}.png".format(save_folder, name, i))
# 定义函数将3D标签转换为2D单通道的png格式标签
def save_label(label, save_folder, name):
label_array = np.array(label.dataobj)
label_array = np.rot90(label_array, 1, axes=(1, 0))
label_array = (label_array - np.min(label_array)) / (np.max(label_array) - np.min(label_array)) * 255
label_array = np.uint8(label_array)
for i in range(label_array.shape[2]):
img = Image.fromarray(label_array[:, :, i])
img = img.convert("L")
img.save("{}/{}_{}.png".format(save_folder, name, i))
# 遍历数据集中的所有图像
for root, dirs, files in os.walk(os.path.join(data_path, "Training Batch 1")):
for name in files:
if name.endswith(".nii"):
image_path = os.path.join(root, name)
# 读取图像
image = nib.load(image_path)
# 对图像进行裁剪
image_array = np.array(image.dataobj)
image_array = np.rot90(image_array, 1, axes=(1, 0))
mask = image_array > 0
coords = np.array(np.nonzero(mask))
x_min, y_min, z_min = coords.min(axis=1)
x_max, y_max, z_max = coords.max(axis=1)
image_array = image_array[x_min:x_max+1, y_min:y_max+1, z_min:z_max+1]
# 对图像进行增强操作
image_array = transform.rotate(image_array, angle=np.random.randint(-10, 10), resize=False, preserve_range=True)
image_array = np.fliplr(image_array) if np.random.random() > 0.5 else image_array
image_array = np.flipud(image_array) if np.random.random() > 0.5 else image_array
image_array = transform.rescale(image_array, scale=np.random.uniform(0.8, 1.2), preserve_range=True)
# 将增强后的图像保存为2D、RGB三通道的png格式图像
save_image(nib.Nifti1Image(image_array, image.affine), os.path.join(save_path, "image"), name[:-4])
# 遍历数据集中的所有标签
for root, dirs, files in os.walk(os.path.join(data_path, "Training Batch 2")):
for name in files:
if name.endswith(".nii"):
label_path = os.path.join(root, name)
# 读取标签
label = nib.load(label_path)
# 对标签进行裁剪
label_array = np.array(label.dataobj)
label_array = np.rot90(label_array, 1, axes=(1, 0))
label_array = label_array[x_min:x_max+1, y_min:y_max+1, z_min:z_max+1]
# 对标签进行增强操作
label_array = transform.rotate(label_array, angle=np.random.randint(-10, 10), resize=False, preserve_range=True)
label_array = np.fliplr(label_array) if np.random.random() > 0.5 else label_array
label_array = np.flipud(label_array) if np.random.random() > 0.5 else label_array
label_array = transform.rescale(label_array, scale=np.random.uniform(0.8, 1.2), preserve_range=True)
# 将增强后的标签保存为2D单通道的png格式标签
save_label(nib.Nifti1Image(label_array, label.affine), os.path.join(save_path, "label"), name[:-4])
```
该代码会遍历LiTS2017数据集中的所有图像和标签,对其进行裁剪、增强和格式转换,并将结果保存到指定的路径。请确保您已经安装了必要的Python库(如nibabel、Pillow和scikit-image)。
相关推荐
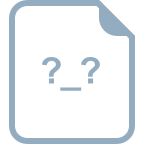
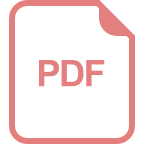









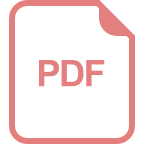
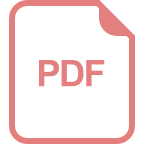
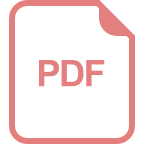