某人从2017年1月1日起“三天打渔两天晒网”,用c语言编写程序求出此人在这输入的某一天中是在打渔还是在晒网,包含平润年
时间: 2024-09-20 15:05:28 浏览: 38
这个题目是一个日期判断的问题,我们需要设计一个简单的C语言程序来确定给定某一天是工作日(打渔)还是休息日(晒网)。假设每年有365天,闰年考虑规则是每4年一闰,但世纪年(如2000年、2100年等)需满足能被400整除才是闰年。
首先,我们需要一个函数来确定给定日期是否是闰年,并计算该年的工作日总数。然后,我们可以跟踪用户输入的每一天,查看它在连续工作日序列中的位置。
下面是一个简单的C语言结构:
```c
#include <stdio.h>
#include <stdbool.h>
// 判断是否是闰年的函数
bool is_leap_year(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
// 计算一年的工作日数
int count_work_days(int year) {
int days = is_leap_year(year) ? 366 : 365;
// 一般工作日假设每周5天,周末休息
days -= (days / 7) * 2; // 减去周六和周日
return days;
}
// 根据工作日序列判断某日是否打渔或晒网
void check_fishing_or_sunbathe(int start_date, int day_to_check) {
int total_days = count_work_days(start_date);
bool fishing = true; // 初始假定在打渔
if (day_to_check > total_days) { // 如果超过总工作日,则超出范围
printf("输入的日期超出了起始日期的一年范围。\n");
return;
}
if (day_to_check % 7 <= 2) { // 打渔日
fishing = true;
} else { // 晒网日
fishing = false;
}
if (fishing) {
printf("这一天是在打渔。\n");
} else {
printf("这一天是在晒网。\n");
}
}
int main() {
int start_date = 2017, day_to_check = ... // 用户输入的具体日期
check_fishing_or_sunbathe(start_date, day_to_check);
return 0;
}
```
阅读全文
相关推荐





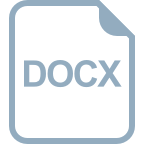

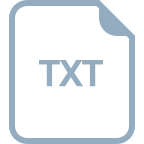
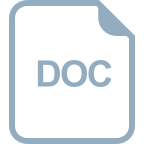


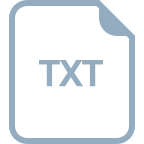
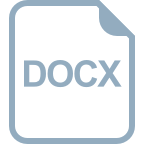
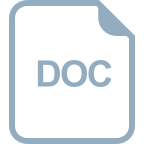
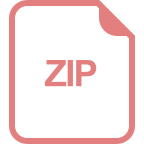
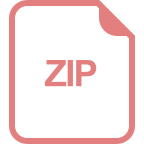