(1)利用DDA算法绘制两条直线,分别是:L1(Point(10, 10), Point(100, 80)), L2(Point(-50, 100), Point(100, -50))代码
时间: 2024-09-24 12:08:25 浏览: 31
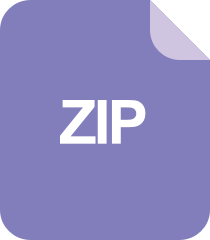
DDA算法绘制直线.zip_DDA算法绘制直线_绘制直线
在Python中,我们可以使用Direct Delta Algorithm (DDA) 来逐像素地绘制二维直线。这里是一个简单的示例,假设我们正在创建一个基本的图形环境,如Pygame或turtle等库:
```python
import pygame
from pygame.locals import *
# 初始化pygame
pygame.init()
# 设置窗口大小
win = pygame.display.set_mode((640, 480))
# 定义两个点
def dda_draw(line):
x1, y1, x2, y2 = line.start.x, line.start.y, line.end.x, line.end.y
dx, dy = abs(x2-x1), abs(y2-y1)
steep = dy > dx # 判断斜率
if steep:
x1, y1 = y1, x1 # 将y轴作为x轴,便于计算
if x1 > x2:
x1, x2 = x2, x1 # 确保起点小于终点
y1, y2 = y2, y1
error = int(dx / 2) # 初始化误差
y = y1
for x in range(x1, x2 + 1):
if steep:
win.set_at((x, y), (255, 0, 0)) # 绘制红色像素
else:
win.set_at((y, x), (255, 0, 0))
error -= dy
if error < 0:
y += 1
error += dx
pygame.display.update()
line1 = DDALine(Point(10, 10), Point(100, 80)) # 创建第一条线实例
line2 = DDALine(Point(-50, 100), Point(100, -50)) # 创建第二条线实例
# 绘制两条线
dda_draw(line1)
dda_draw(line2)
# 游戏主循环
run = True
while run:
for event in pygame.event.get():
if event.type == QUIT:
run = False
pygame.quit()
```
在这个例子中,`DDALine`是一个自定义类,用于封装直线的信息,并提供按照DDA算法进行绘制的方法。
请注意,这个例子没有包括`DDALine`类的具体实现,因为这通常涉及到更复杂的数学处理和边界检查。上面的代码仅给出了基本的概念和流程。如果你需要完整实现,你需要自己添加这个类以及相关的DDA算法步骤。
阅读全文
相关推荐
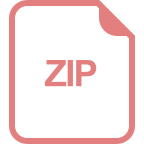
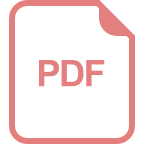






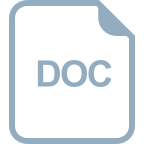
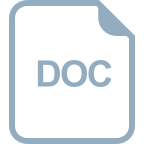
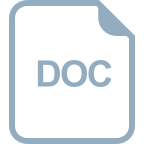
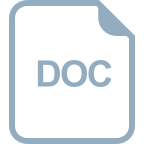



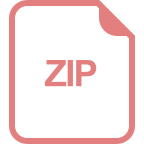