用python 利用DDA算法绘制两条直线,分别是:L1(Point(10, 10), Point(100, 80)), L2(Point(-50, 100), Point(100, -50))。
时间: 2024-09-22 10:09:20 浏览: 72
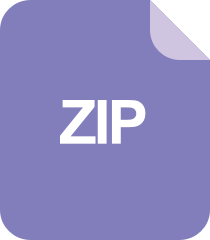
DDA算法绘制直线.zip_DDA算法绘制直线_绘制直线
在Python中,我们可以使用双精度逼近法(Double Dilation Algorithm, 简称DDA)来近似绘制二维直线上的一系列点。这个算法主要用于计算机图形学中的基本绘图操作。以下是利用DDA绘制给定直线L1和L2的步骤:
首先,我们需要导入必要的数学库,并定义一个辅助函数来计算每个点的坐标。这里我们假设步长是一个整数,例如1。
```python
import math
def dda_draw(line_start, line_end, step):
x1, y1 = line_start.x, line_start.y
x2, y2 = line_end.x, line_end.y
dx = x2 - x1
dy = y2 - y1
is_steep = abs(dy) > abs(dx)
# 如果斜率大于0,则从左上角开始画
if is_steep:
x1, y1 = y1, x1
x2, y2 = y2, x2
dx, dy = dy, dx
length = max(abs(dx), abs(dy))
xx, yy = x1, y1
for i in range(int(length + step)):
yield (xx, yy)
if is_steep:
xx += step * (-1 if dx < 0 else 1)
else:
yy += step * (-1 if dy < 0 else 1)
# 定义线段L1和L2
line1_start = Point(10, 10)
line1_end = Point(100, 80)
line2_start = Point(-50, 100)
line2_end = Point(100, -50)
# 使用步长绘制两条线,比如1像素为单位
for i, point in enumerate(dda_draw(line1_start, line1_end, 1)):
print(f"Point {i}: ({point[0]}, {point[1]})")
for i, point in enumerate(dda_draw(line2_start, line2_end, 1)):
print(f"Point {i}: ({point[0]}, {point[1]})")
```
请注意,`Point`类需要你自己定义,因为它在这里并未提供。你可以创建一个简单的类来表示点,如:
```python
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
```
阅读全文
相关推荐
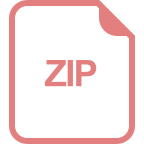
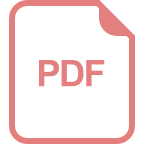







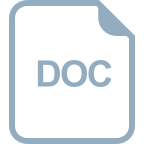
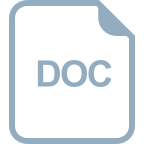
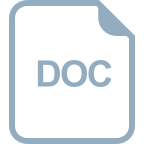


