ostream &operator<<(ostream &out,m_class &m) { out <<"name"<<m.name<<"age"<<m.age<<endl; return out; }
时间: 2024-06-13 10:05:59 浏览: 129
这是一个C++中的重载运算符<<的例子,用于将自定义类m_class的对象输出到流中。其中,out是一个ostream类型的输出流对象,m是一个m_class类型的对象。该函数的实现将m_class对象的成员变量name和age输出到out流中,并在最后加上一个换行符endl,最后返回out流对象。可以通过在程序中使用cout来输出m_class对象,例如:cout << m_obj; 其中m_obj是一个m_class类型的对象。
相关问题
#include <iostream> #include <string> using namespace std; class Point { public: string name; double x; double y; friend ostream& operator<<(ostream& os, const Point& p); }; ostream& operator<<(ostream& os, const Point& p) { os << p.name << "(" << p.x << "," << p.y << ")"; return os; } int main() { Point pt={2,3}; cout << pt << endl; return 0; }如何绘制点与点之间的相对位置
在这个程序中,我们定义了一个表示二维点的类 Point,其中包含了点的名称和 x,y 坐标。我们还重载了输出运算符 <<,使得可以方便地输出 Point 对象。
如果想要绘制点与点之间的相对位置,需要使用图形库或者绘图软件。以下是使用 Qt 图形库绘制的示例代码:
```
#include <QtGui>
class Point {
public:
QString name;
double x;
double y;
};
class MyWidget : public QWidget {
public:
MyWidget(QWidget *parent = nullptr) : QWidget(parent) {
setFixedSize(400, 400);
}
void paintEvent(QPaintEvent *event) override {
Q_UNUSED(event);
QPainter painter(this);
painter.setRenderHint(QPainter::Antialiasing);
// 设置点的坐标
Point p1 = {"A", 100, 100};
Point p2 = {"B", 200, 200};
Point p3 = {"C", 300, 100};
// 绘制点
painter.setPen(Qt::black);
painter.drawEllipse(p1.x - 3, p1.y - 3, 6, 6);
painter.drawEllipse(p2.x - 3, p2.y - 3, 6, 6);
painter.drawEllipse(p3.x - 3, p3.y - 3, 6, 6);
// 绘制点之间的相对位置
painter.setPen(Qt::red);
painter.drawLine(p1.x, p1.y, p2.x, p2.y);
painter.drawLine(p2.x, p2.y, p3.x, p3.y);
painter.drawLine(p3.x, p3.y, p1.x, p1.y);
}
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
MyWidget widget;
widget.show();
return app.exec();
}
```
在上述代码中,我们定义了一个继承自 QWidget 的 MyWidget 类,并重载了它的 paintEvent 函数,在此函数中使用 QPainter 绘制出点和线段。通过设置 QPen 的颜色和线宽,可以实现不同的效果。
ostream & operator<<(ostream& cout,Person& p)
这是一个重载输出流运算符的函数,它的作用是将一个 Person 类型的对象输出到标准输出流中。具体实现方式可以参考以下代码:
```c++
#include<iostream>
#include<string>
using namespace std;
class Person{
public:
Person(string name,int age):m_name(name),m_age(age){}
friend ostream & operator<<(ostream& cout,Person& p);
private:
string m_name;
int m_age;
};
ostream & operator<<(ostream& cout,Person& p){
cout<<"Name: "<<p.m_name<<", Age: "<<p.m_age<<endl;
return cout;
}
int main(){
Person p("Tom", 18);
cout << p; // 输出 "Name: Tom, Age: 18"
return 0;
}
```
在上述代码中,重载输出流运算符的函数 `operator<<` 被定义为 `Person` 类的友元函数,它可以直接访问 `Person` 类的私有成员变量 `m_name` 和 `m_age`,并将它们输出到标准输出流中。在主函数中,我们创建一个 `Person` 对象 `p`,然后通过 `cout << p` 的方式将其输出到标准输出流中。
阅读全文
相关推荐
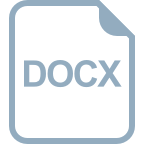
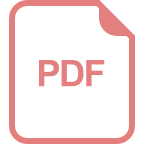








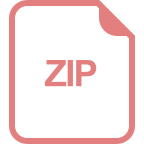
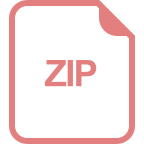
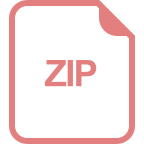
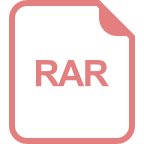