#include <iostream> #include <string> using namespace std; class Point { protected: string type; double x, y; public: Point() : type("Point"), x(0), y(0) {} Point(double x, double y) : type("Point"), x(x), y(y) {} virtual ~Point() { cout << type << " object is destroyed." << endl; } friend ostream& operator<<(ostream& os, const Point& p) { os << "Type: " << p.type << endl; os << "Coordinates: (" << p.x << ", " << p.y << ")" << endl; return os; } virtual void PrintName() { cout << "Type: " << type << endl; } }; class Circle : public Point { protected: double r; public: Circle() : Point(), r(0) { type = "Circle"; } Circle(double x, double y, double r) : Point(x, y), r(r) { type = "Circle"; } virtual ~Circle() { cout << type << " object is destroyed." << endl; } friend ostream& operator<<(ostream& os, const Circle& c) { os << static_cast<const Point&>(c); os << "Radius: " << c.r << endl; return os; } virtual void PrintName() { cout << "Type: " << type << endl; } }; class Cylinder : public Circle { protected: double h; public: Cylinder() : Circle(), h(0) { type = "Cylinder"; } Cylinder(double x, double y, double r, double h) : Circle(x, y, r), h(h) { type = "Cylinder"; } virtual ~Cylinder() { cout << type << " object is destroyed." << endl; } friend ostream& operator<<(ostream& os, const Cylinder& cy) { os << static_cast<const Circle&>(cy); os << "Height: " << cy.h << endl; return os; } virtual void PrintName() { cout << "Type: " << type << endl; } }; int main() { Point p1(1, 2); cout << p1 << endl; Circle c1(1, 2, 3); cout << c1 << endl; Cylinder cy1(1, 2, 3, 4); cout << cy1 << endl; Point* p; p = &p1; p->PrintName(); p = &c1; p->PrintName(); p = &cy1; p->PrintName(); return 0; } 写出该程序的大致流程
时间: 2024-04-25 10:25:58 浏览: 51
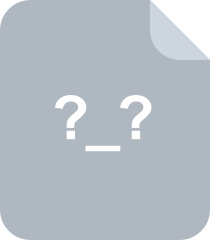
#include <iostream>
1. 引入iostream和string头文件。
2. 声明Point类,包含类型(type)和坐标(x和y)属性,有默认构造函数和带参数构造函数,定义了一个友元函数用于输出对象信息,定义了一个虚函数PrintName用于输出对象类型。
3. 声明Circle类,继承自Point类,增加了半径(r)属性,有默认构造函数和带参数构造函数,定义了一个友元函数用于输出对象信息,重写了虚函数PrintName用于输出对象类型。
4. 声明Cylinder类,继承自Circle类,增加了高度(h)属性,有默认构造函数和带参数构造函数,定义了一个友元函数用于输出对象信息,重写了虚函数PrintName用于输出对象类型。
5. 在main函数中,分别创建一个Point对象、一个Circle对象和一个Cylinder对象,输出它们的信息。
6. 声明一个指向Point对象的指针p,在分别将p指向Point对象、Circle对象和Cylinder对象后,分别调用p的PrintName函数,输出对象类型。
7. 程序结束,析构Point、Circle和Cylinder对象。
阅读全文
相关推荐









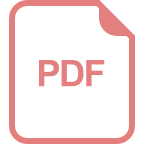