apache图片裁剪
时间: 2024-08-27 13:00:39 浏览: 48
Apache Commons Imaging是一个流行的开源库,用于处理各种图像文件格式,并提供了一些方便的功能,包括图片的裁剪。在Java中,你可以使用它来裁剪图片。这里是一个简单的示例:
```java
import org.apache.commons.imaging.ImageIO;
import org.apache.commons.imaging.util.IoUtils;
import javax.imageio.ImageReader;
import javax.imageio.stream.ImageInputStream;
import java.awt.geom.Point2D;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
public class ImageCropExample {
public static BufferedImage cropImage(File source, Point2D destPoint, int width, int height) throws IOException {
File croppedFile = new File(source.getName() + "_cropped.jpg");
BufferedImage originalImage = ImageIO.read(source);
int x = (int) destPoint.getX();
int y = (int) destPoint.getY();
int srcWidth = originalImage.getWidth();
int srcHeight = originalImage.getHeight();
// 裁剪边界校验
if (x < 0 || y < 0 || x > srcWidth - width || y > srcHeight - height) {
throw new IllegalArgumentException("Invalid cropping coordinates");
}
BufferedImage croppedBufferedImage = originalImage.getSubimage(x, y, width, height);
try (ImageOutputStream outputStream = ImageIO.createImageOutputStream(croppedFile)) {
ImageIO.write(croppedBufferedImage, "jpg", outputStream);
}
return croppedBufferedImage;
}
public static void main(String[] args) {
try {
BufferedImage croppedImage = cropImage(new File("source.jpg"), new Point2D.Float(50f, 75f), 200, 150);
// 对裁剪后的图片做进一步操作...
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这个例子中,我们首先读取源图片,然后指定裁剪点和尺寸来创建一个新的`BufferedImage`对象,最后将裁剪后的图像保存到新的文件中。
阅读全文
相关推荐




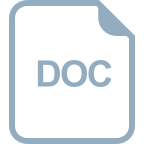













