import torch tensor=torch.tensor([2,1,4,3],[1,2,3,4],[4,3,2,1]) print(tensor)这段代码有什么错误
时间: 2024-11-06 07:21:10 浏览: 33
这段代码的目的是使用PyTorch库将输入的数据转换为张量,并打印出来。然而,有一个语法错误导致了`TypeError`。在`torch.tensor()`函数中,当你试图一次性传入三个列表作为参数,而实际上这个函数期望的是一个或多个一维或二维的张量数据。
正确的做法应该是将这三层嵌套的列表合并为一个单一的二维或三维张量。这里我们假设你想创建一个三维张量,应该这样做:
```python
import torch
# 将三个列表拼接在一起形成一个三维张量的每个维度
tensor = torch.tensor([list(range(4))] * 3)
# 或者更明确地定义每个维度的大小
tensor = torch.tensor([2, 1, 4, 3], [1, 2, 3, 4], [4, 3, 2, 1])
print(tensor)
```
如果只想得到一个二维张量,你可以去掉嵌套的列表结构:
```python
tensor = torch.tensor([[2, 1, 4, 3], [1, 2, 3, 4], [4, 3, 2, 1]])
print(tensor)
```
现在,这两段代码都不会抛出`TypeError`,并且会打印出相应的张量结果。
相关问题
face_tensor = face_tensor.type('torch.FloatTensor')
这行代码是将变量 `face_tensor` 的数据类型转换为 `torch.FloatTensor` 类型。
在 PyTorch 中,每个 tensor(张量)都有一个数据类型(data type),它定义了 tensor 中元素的类型。`torch.FloatTensor` 类型表示一个 32 位浮点数的 tensor,即每个元素占用 4 个字节的空间。
该行代码的作用可能是将 `face_tensor` 的数据类型从其他类型(如 `torch.DoubleTensor`)转换为 `torch.FloatTensor`,以便它可以与其他的 `torch.FloatTensor` 类型的 tensor 进行操作。
import torch a = torch.tensor([2, 3, 4]) b = torch.tensor([2, 3, 4]) c =torch.add(a,b) print(c)
The output of this code will be:
tensor([4, 6, 8])
This is because we are adding two tensors a and b element-wise using the torch.add() function, and the resulting tensor c contains the sum of each corresponding element in a and b. In other words, c[i] = a[i] + b[i] for each i in the range of the tensor dimensions.
阅读全文
相关推荐
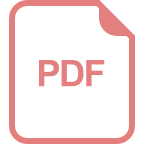
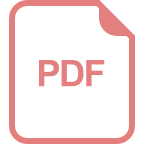
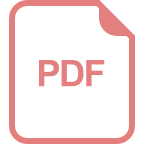














