用python写一段代码,要求如下:遍历一个文件夹下的所有文件,每个文件有若干图片,每个文件下的图片分别镜像翻转,向左旋转90度,向右旋转90度,获得的图片按照最初的文件夹的格式保存下来,每个文件夹有若干存储图像的文件夹,
时间: 2024-10-11 19:05:52 浏览: 26
在Python中,我们可以使用`os`, `PIL`(Python Imaging Library)以及其后续项目`Pillow`库来完成这个任务。下面是一个简单的示例代码:
```python
from PIL import Image
import os
# 定义处理图片的方法
def process_image(file_path):
with Image.open(file_path) as img:
# 镜像翻转
flipped_img = img.transpose(Image.FLIP_LEFT_RIGHT)
# 左旋90度
rotated_left_img = flipped_img.rotate(90, expand=True)
# 右旋90度
rotated_right_img = flipped_img.rotate(-90, expand=True)
# 生成新的文件名,并保存到原文件夹下
new_file_name = os.path.splitext(os.path.basename(file_path))[0] + "_processed" + os.path.splitext(file_path)[1]
processed_path = os.path.join(os.path.dirname(file_path), new_file_name)
rotated_right_img.save(processed_path)
# 遍历指定目录及其所有子目录下的图片
def traverse_and_process_images(root_dir):
for root, dirs, files in os.walk(root_dir):
for file in files:
if file.lower().endswith(('.jpg', '.jpeg', '.png', '.gif')): # 只处理常见的图片格式
image_path = os.path.join(root, file)
process_image(image_path)
# 调用函数开始处理
traverse_and_process_images('/path/to/your/directory')
```
在这个代码中,你需要将`'/path/to/your/directory'`替换为你实际要处理的文件夹路径。注意,这个脚本会覆盖原始图片并创建镜像和旋转后的版本。
阅读全文
相关推荐
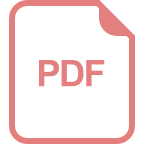
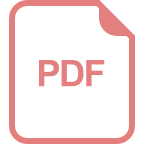
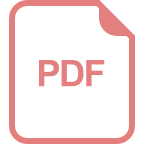




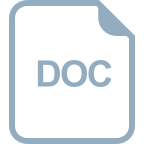
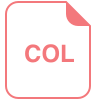
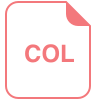
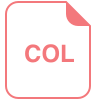
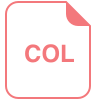
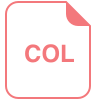
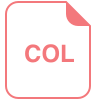
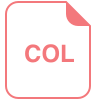
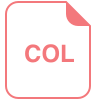
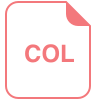
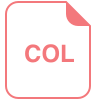

