解释这行代码files = dir(fullfile(oriFolderPath, '*.pdf'));
时间: 2024-04-12 21:33:10 浏览: 139
这行代码使用了MATLAB的dir函数,用于列出指定文件夹中满足特定条件的文件。具体解释如下:
- fullfile(oriFolderPath, '*.pdf'):使用fullfile函数将 oriFolderPath 和 '*.pdf' 这两个参数合并为一个完整的路径。其中,oriFolderPath 是一个文件夹路径,而 '*.pdf' 是一个通配符,表示匹配所有以 .pdf 结尾的文件。
- dir(fullfile(oriFolderPath, '*.pdf')):调用dir函数,传入完整的路径作为参数,返回一个结构体数组。这个结构体数组包含了 oriFolderPath 文件夹中所有以 .pdf 结尾的文件的相关信息。
- files = dir(fullfile(oriFolderPath, '*.pdf')):将返回的结构体数组赋值给变量 files,以便后续对文件进行操作。
总之,这行代码的作用是在 oriFolderPath 文件夹中查找所有以 .pdf 结尾的文件,并将它们的信息存储在 files 变量中供后续使用。
相关问题
解释以下每一行代码% 指定文件夹路径 oriFolderPath = 'path/to/ori'; subFolderPath = 'path/to/zuoye/sub'; % 创建 sub 文件夹 mkdir(subFolderPath); % 获取 ori 文件夹中的所有 pdf 文件 files = dir(fullfile(oriFolderPath, '*.pdf')); % 遍历每个 pdf 文件 for i = 1:numel(files) % 获取当前 pdf 文件名和路径 pdfName = files(i).name; pdfPath = fullfile(oriFolderPath, pdfName); % 提取 pdf 编号 [~, fileName, ~] = fileparts(pdfName); folderName = fileName; % 在 sub 文件夹中创建以编号命名的子文件夹 subFolder = fullfile(subFolderPath, folderName); mkdir(subFolder); % 移动 pdf 文件到对应编号的子文件夹中,并重命名为 report.pdf newPdfName = 'report.pdf'; newPdfPath = fullfile(subFolder, newPdfName); movefile(pdfPath, newPdfPath); end % 删除 sub 文件夹及其内容 rmdir(subFolderPath, 's');
这段代码是用来操作文件夹和文件的。我将逐行解释每一行代码的作用。
```matlab
oriFolderPath = 'path/to/ori';
subFolderPath = 'path/to/zuoye/sub';
```
这两行代码用来指定文件夹的路径。`oriFolderPath` 是原始文件夹的路径,`subFolderPath` 是子文件夹的路径。
```matlab
mkdir(subFolderPath);
```
这一行代码用来创建子文件夹。它使用 `mkdir` 函数来创建一个新的文件夹,文件夹的路径由 `subFolderPath` 指定。
```matlab
files = dir(fullfile(oriFolderPath, '*.pdf'));
```
这一行代码用来获取原始文件夹中所有以 `.pdf` 结尾的文件。`dir` 函数根据指定的路径和文件筛选条件返回一个结构体数组,其中包含了满足条件的文件信息。
```matlab
for i = 1:numel(files)
```
这一行代码开始了一个循环,用来遍历每个 pdf 文件。`numel` 函数返回数组的元素个数。
```matlab
pdfName = files(i).name;
pdfPath = fullfile(oriFolderPath, pdfName);
```
这两行代码用来获取当前 pdf 文件的名称和完整路径。`files(i).name` 获取了第 i 个文件的名称,`fullfile` 函数根据指定的文件夹路径和文件名生成完整的文件路径。
```matlab
[~, fileName, ~] = fileparts(pdfName);
folderName = fileName;
```
这两行代码用来提取 pdf 文件的编号。`fileparts` 函数可以将文件名分解为文件路径、文件名和扩展名。在这里,我们只需要文件名部分,所以用 `~` 来忽略掉不需要的部分。
```matlab
subFolder = fullfile(subFolderPath, folderName);
mkdir(subFolder);
```
这两行代码用来在子文件夹中创建以编号命名的子文件夹。`fullfile` 函数用来生成子文件夹的完整路径,`mkdir` 函数用来创建子文件夹。
```matlab
newPdfName = 'report.pdf';
newPdfPath = fullfile(subFolder, newPdfName);
movefile(pdfPath, newPdfPath);
```
这三行代码用来将 pdf 文件移动到对应编号的子文件夹中,并重命名为 `report.pdf`。`movefile` 函数用来移动文件并重命名。
```matlab
rmdir(subFolderPath, 's');
```
这一行代码用来删除子文件夹及其内容。`rmdir` 函数用来删除文件夹,`'s'` 参数表示要删除文件夹及其内容。
这就是这段代码的解释了。它的主要功能是将原始文件夹中的 pdf 文件按照编号移动到子文件夹中,并重命名为 `report.pdf`。最后,删除子文件夹及其内容。
优化代码% 指定文件夹路径 oriFolderPath = 'path/to/ori'; subFolderPath = 'path/to/zuoye/sub'; % 创建 sub 文件夹 mkdir(subFolderPath); % 获取 ori 文件夹中的所有 pdf 文件 files = dir(fullfile(oriFolderPath, '*.pdf')); % 遍历每个 pdf 文件 for i = 1:length(files) % 获取当前 pdf 文件名和路径 pdfName = files(i).name; pdfPath = fullfile(oriFolderPath, pdfName); % 提取 pdf 编号 [~, fileName, ~] = fileparts(pdfName); folderName = fileName; % 在 sub 文件夹中创建以编号命名的子文件夹 subFolder = fullfile(subFolderPath, folderName); mkdir(subFolder); % 移动 pdf 文件到对应编号的子文件夹中,并重命名为 report.pdf newPdfName = 'report.pdf'; newPdfPath = fullfile(subFolder, newPdfName); movefile(pdfPath, newPdfPath); end % 删除 sub 文件夹及其内容 rmdir(subFolderPath, 's');
您的代码看起来已经很不错了,但我可以提供一些建议来优化它:
```matlab
oriFolderPath = 'path/to/ori';
subFolderPath = 'path/to/zuoye/sub';
% 创建 sub 文件夹
if ~exist(subFolderPath, 'dir')
mkdir(subFolderPath);
end
% 获取 ori 文件夹中的所有 pdf 文件
filePattern = fullfile(oriFolderPath, '*.pdf');
pdfFiles = dir(filePattern);
% 遍历每个 pdf 文件
for i = 1:length(pdfFiles)
% 获取当前 pdf 文件名和路径
pdfName = pdfFiles(i).name;
pdfPath = fullfile(oriFolderPath, pdfName);
% 提取 pdf 编号
[~, fileName, ~] = fileparts(pdfName);
folderName = fileName;
% 在 sub 文件夹中创建以编号命名的子文件夹
subFolder = fullfile(subFolderPath, folderName);
if ~exist(subFolder, 'dir')
mkdir(subFolder);
end
% 移动 pdf 文件到对应编号的子文件夹中,并重命名为 report.pdf
newPdfName = 'report.pdf';
newPdfPath = fullfile(subFolder, newPdfName);
movefile(pdfPath, newPdfPath);
end
% 删除 sub 文件夹及其内容
rmdir(subFolderPath, 's');
```
这里是一些对代码进行改进的地方:
1. 添加了对 `subFolderPath` 和 `subFolder` 是否存在的检查,如果不存在则创建。这样可以避免在多次运行代码时出现错误。
2. 使用更具描述性的变量名 `pdfFiles` 替代 `files`,使代码更易读。
3. 使用 `filePattern` 替代 `fullfile(oriFolderPath, '*.pdf')`,更清晰地表示要匹配的文件类型。
4. 使用 `~exist(subFolder, 'dir')` 来检查文件夹是否存在,如果不存在则创建文件夹。这样可以避免在多次运行代码时出现错误。
5. 添加了对 `subFolderPath` 是否存在的检查,如果存在则删除该文件夹及其内容,以便重新创建新的子文件夹。
这些改进可以提高代码的可靠性和可读性。希望对您有所帮助!
阅读全文
相关推荐
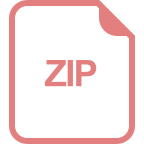
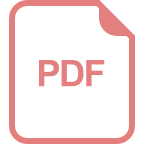
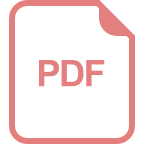














