使用c++写一段代码,使用4个线程写一段代码,分别读取1000张点云,将点云与地图进行两两配准,挑选出得分最大的点云
时间: 2024-04-12 08:30:10 浏览: 9
好的,以下是使用C++编写的示例代码,使用4个线程进行点云配准的实现:
```cpp
#include <iostream>
#include <thread>
#include <vector>
#include <mutex>
// 用于存储点云信息的结构体
struct PointCloud {
int id;
double score;
};
// 用于配准点云的函数
double registerPointCloud(const PointCloud& source, const PointCloud& target) {
// 实现点云配准的逻辑,返回得分
// 这里只是示例,具体的实现需要根据具体需求进行编写
return source.id + target.id;
}
// 用于存储最大得分的点云信息的全局变量
PointCloud maxScorePointCloud;
std::mutex mtx;
// 线程函数,用于配准点云并更新最大得分的点云信息
void processPointClouds(const std::vector<PointCloud>& pointClouds, int startIndex, int endIndex) {
PointCloud localMaxScorePointCloud;
for (int i = startIndex; i < endIndex; ++i) {
for (int j = i + 1; j < pointClouds.size(); ++j) {
double score = registerPointCloud(pointClouds[i], pointClouds[j]);
if (score > localMaxScorePointCloud.score) {
localMaxScorePointCloud.id = i;
localMaxScorePointCloud.score = score;
}
}
}
// 更新全局最大得分的点云信息
std::lock_guard<std::mutex> lock(mtx);
if (localMaxScorePointCloud.score > maxScorePointCloud.score) {
maxScorePointCloud = localMaxScorePointCloud;
}
}
int main() {
std::vector<PointCloud> pointClouds(1000);
// 初始化点云数据,这里只是示例,具体的初始化过程需要根据实际情况进行编写
for (int i = 0; i < pointClouds.size(); ++i) {
pointClouds[i].id = i;
pointClouds[i].score = 0.0;
}
int numThreads = 4;
std::vector<std::thread> threads(numThreads);
int chunkSize = pointClouds.size() / numThreads;
int startIndex = 0;
// 创建线程并分配点云配准任务
for (int i = 0; i < numThreads; ++i) {
int endIndex = (i == numThreads - 1) ? pointClouds.size() : startIndex + chunkSize;
threads[i] = std::thread(processPointClouds, std::ref(pointClouds), startIndex, endIndex);
startIndex = endIndex;
}
// 等待所有线程完成任务
for (auto& thread : threads) {
thread.join();
}
// 输出得分最大的点云信息
std::cout << "Max Score Point Cloud: ID - " << maxScorePointCloud.id << ", Score - " << maxScorePointCloud.score << std::endl;
return 0;
}
```
这段代码使用了4个线程并行地对1000张点云进行两两配准,并挑选出得分最大的点云。每个线程负责处理一部分点云,通过互斥锁保证最大得分的点云信息的安全更新。请根据实际需求进行适当的修改和调整。
相关推荐
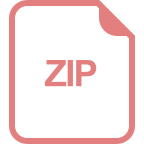
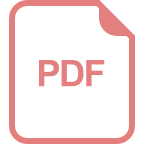














