python推箱子代码
时间: 2024-09-10 07:16:04 浏览: 56
Python编写推箱子(Puzzle Game, 如经典的 Sokoban)的代码通常涉及搜索算法(如A*、广度优先搜索或深度优先搜索)、游戏规则的理解以及数据结构的运用。下面是一个简单的基于命令行交互的Sokoban游戏示例:
```python
import heapq
# 定义方块、玩家和目标位置
class Block:
pass
class Player:
def __init__(self, pos):
self.pos = pos
class Goal:
pass
# 游戏地图表示
def create_board(size):
board = [[Block() for _ in range(size)] for _ in range(size)]
# 初始化一些随机位置的方块
for _ in range(4):
x, y = random.randint(0, size-1), random.randint(0, size-1)
if board[x][y] is not None:
continue
board[x][y] = Block()
return board
# A*搜索算法简化版
def a_star_search(board, player, goal, heuristic_func):
open_set = [(0, (player.pos,))]
came_from = {}
g_score = {player.pos: 0}
f_score = {player.pos: heuristic_func(player.pos, goal)}
while open_set:
current_pos, path = heapq.heappop(open_set)
if current_pos == goal:
return path
for neighbor in get_neighbors(board, current_pos):
tentative_g_score = g_score[current_pos] + 1
if neighbor not in g_score or tentative_g_score < g_score[neighbor]:
g_score[neighbor] = tentative_g_score
f_score[neighbor] = tentative_g_score + heuristic_func(neighbor, goal)
came_from[neighbor] = current_pos
heapq.heappush(open_set, (f_score[neighbor], (neighbor,)))
return None
# 边缘判断和邻居获取函数
def get_neighbors(board, pos):
x, y = pos
neighbors = []
directions = [(1, 0), (-1, 0), (0, 1), (0, -1)]
for dx, dy in directions:
new_x, new_y = x + dx, y + dy
if 0 <= new_x < len(board) and 0 <= new_y < len(board[0]) and board[new_x][new_y] is None:
neighbors.append((new_x, new_y))
return neighbors
# 示例游戏主循环
def play_game():
board = create_board(10)
player = Player((5, 5)) # 玩家初始位置
goal = Goal((9, 9)) # 目标位置
path = a_star_search(board, player, goal, lambda p, g: manhattan_distance(p, g)) # 使用曼哈顿距离作为启发式函数
if path:
print("找到了路径:", path)
move_player_to_path(board, player, path)
else:
print("无解,无法到达目标")
# ... 其他辅助函数比如移动玩家等 ...
```
在这个例子中,我们首先定义了游戏元素类,然后实现了A*搜索算法来寻找从起点到终点的最短路径。`play_game()`函数是游戏的核心,它创建了一个地图,设置好玩家和目标位置,然后调用搜索函数找到路径。
请注意这只是一个基础版本,实际的游戏可能会更复杂,包括图形用户界面、障碍物处理、游戏循环控制等功能。
阅读全文
相关推荐
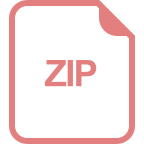
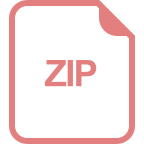
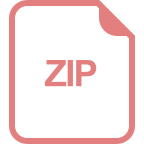













