用c++写出上述代码,代码越长越好,越基础越好。
时间: 2024-11-20 20:42:29 浏览: 6
当然,这是一个基础版本的C++代码实现,使用了while循环来逐个处理输入的字符串:
```cpp
#include <iostream>
#include <string>
#include <stack>
using namespace std;
int main() {
stack<int> scores; // 使用栈来模拟撤销操作
int current_score = 0; // 当前得分
string input;
while (cin >> input) { // 读取输入直到结束
if (isdigit(input[0])) { // 如果是整数
current_score = stoi(input); // 转换为整数并添加到得分
} else if (input == "+") { // 如果是+
if (!scores.empty()) { // 取出最近的得分
current_score += scores.top();
scores.pop(); // 移除最后一个元素
}
} else if (input == "D") { // 如果是D
if (!scores.empty()) { // 取出最近的得分
int prev_score = scores.top();
scores.pop();
current_score += prev_score * 2;
}
} else if (input == "C") { // 如果是C
while (!scores.empty()) { // 清空栈,直至找到有效得分
scores.pop();
}
}
scores.push(current_score); // 添加当前得分到栈,以备后续C操作
cout << "Round Score: " << current_score << endl;
}
int total_points = 0;
while (!scores.empty()) { // 计算所有有效得分的总和
total_points += scores.top();
scores.pop();
}
cout << "Total Points: " << total_points << endl;
return 0;
}
```
注意:这个代码没有使用类和模板,直接通过基本的数据结构(栈)来实现,每个操作都对应了一个单独的if-else分支,虽然简洁但也相对复杂,不太符合现代C++的风格。在实际项目中,可能会考虑封装成更优雅的函数或类来管理得分状态。
阅读全文
相关推荐
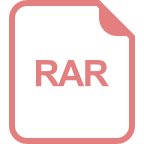
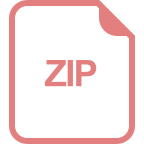
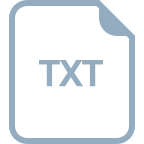


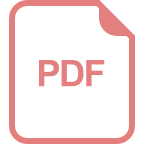
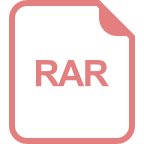
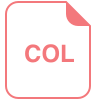
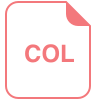
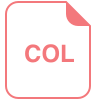
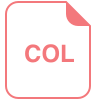
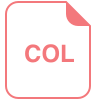





