javafx读取Outlook邮件
时间: 2023-08-11 18:05:14 浏览: 184
你可以使用JavaMail API来读取Outlook邮件。下面是一个简单的示例代码,演示如何使用JavaMail API连接到Outlook的POP3或IMAP服务器并读取邮件。
```java
import java.util.Properties;
import javax.mail.*;
public class OutlookEmailReader {
public static void main(String[] args) {
// 邮箱配置
String host = "pop-mail.outlook.com"; // 或者使用 "imap-mail.outlook.com" for IMAP
String username = "your-email@example.com";
String password = "your-password";
// 邮箱属性
Properties properties = new Properties();
properties.put("mail.store.protocol", "pop3"); // 或者使用 "imap" for IMAP
properties.put("mail.pop3.host", host);
properties.put("mail.pop3.port", "995");
properties.put("mail.pop3.starttls.enable", "true");
try {
// 创建会话
Session session = Session.getDefaultInstance(properties);
// 连接到邮箱
Store store = session.getStore();
store.connect(host, username, password);
// 打开收件箱文件夹
Folder inbox = store.getFolder("INBOX");
inbox.open(Folder.READ_ONLY);
// 读取邮件
Message[] messages = inbox.getMessages();
for (Message message : messages) {
System.out.println("Subject: " + message.getSubject());
System.out.println("From: " + message.getFrom()[0]);
System.out.println("Text: " + message.getContent().toString());
}
// 关闭连接
inbox.close(false);
store.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
在上述代码中,你需要将`host`、`username`和`password`替换为你的Outlook邮箱的相关信息。代码中使用了POP3协议来连接到Outlook邮箱,如果你想使用IMAP协议,只需将相应的属性设置为IMAP相关的参数即可。
请确保在运行代码之前,你已经添加了JavaMail API的依赖。你可以从Maven中央仓库获取JavaMail API的最新版本。
希望这可以帮助到你读取Outlook邮件!如有任何问题,请随时提问。
阅读全文
相关推荐
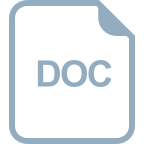
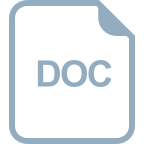
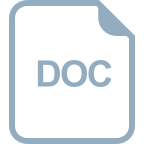
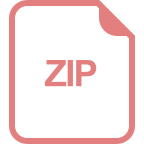
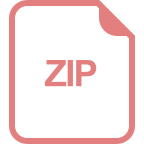
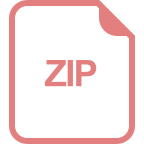
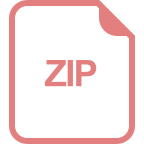
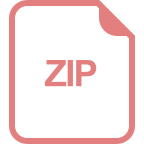
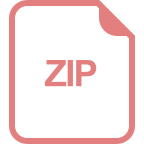
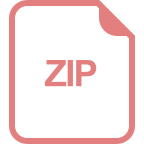
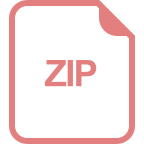
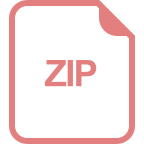
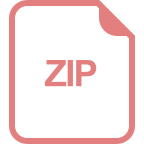
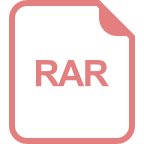