帮我用 c语言写写一个函数可以读入n(0<n<100)个整数,并求出这n个整数中的最大值。 此题要求递归求解,且不允许使用全局变量。在此题的所有提交中只要出现非递归提交,无论其他提交是否是递归求解此题均为0分。
时间: 2024-12-20 13:22:38 浏览: 6
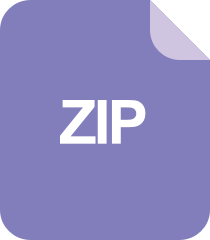
c代码-10个整数找最xiap值
当然,你可以创建一个名为 `findMaxRecursively` 的递归函数来解决这个问题。由于题目要求不使用全局变量,我们可以传递一个额外的参数 `currentMax` 来保存当前已知的最大值。以下是对应的 C 代码:
```c
#include <stdio.h>
// 函数声明,形参 currentMax 用于记录当前已知的最大值
int findMaxRecursively(int n, int currentMax);
int main() {
int n;
printf("请输入一个0到99之间的整数n: ");
scanf("%d", &n);
if (n < 0 || n > 99) {
printf("输入的数值超出范围,请重新输入。\n");
return 0;
}
// 调用 findMaxRecursively 函数,传入初始最大值为0
int result = findMaxRecursively(n, 0);
printf("给定 %d 个整数中的最大值是: %d\n", n, result);
return 0;
}
// 递归函数实现
int findMaxRecursively(int n, int currentMax) {
if (n == 1) { // 递归基本情况:只剩下一个数
return currentMax;
} else {
int nextInput;
scanf("%d", &nextInput); // 读取下一个整数
currentMax = (nextInput > currentMax) ? nextInput : currentMax; // 更新最大值
return findMaxRecursively(n - 1, currentMax); // 递归调用,n 减一继续寻找最大值
}
}
```
在这个程序中,用户会被提示输入一个介于 0 和 99 之间的整数,然后递归地读取每个整数并更新最大值,直到所有的数都考虑过为止。
阅读全文
相关推荐
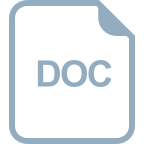
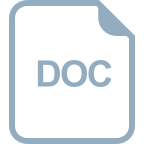
















