Java,已知url和请求体id,key,对请求体进行aes加密,有aesutil工具,发送post请求获取数据的代码怎么写
时间: 2024-10-11 14:14:12 浏览: 25
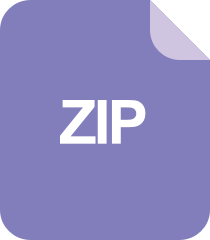
Java Https工具类,Java Https Post请求

在Java中,你可以使用`AESUtil`工具类配合`HttpURLConnection`或者第三方库如`OkHttp`或`Apache HttpClient`来进行AES加密的POST请求。这里提供一个基本的例子,假设`AESUtil`已经实现了AES加解密功能:
```java
import javax.crypto.Cipher;
import java.nio.charset.StandardCharsets;
import java.util.Base64;
public class AESPostRequestExample {
private static final String URL = "http://example.com/api";
private static final String REQUEST_ID = "request_id";
private static final String KEY = "your_secret_key";
// 假设AESUtil有这样的方法来加密和解密
private static String encrypt(String data, String key) {
try {
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, getSecretKeySpec(key));
byte[] encryptedBytes = cipher.doFinal(data.getBytes(StandardCharsets.UTF_8));
return Base64.getEncoder().encodeToString(encryptedBytes);
} catch (Exception e) {
throw new RuntimeException("Error while encrypting", e);
}
}
private static SecretKeySpec getSecretKeySpec(String key) throws NoSuchAlgorithmException {
return new SecretKeySpec(key.getBytes(), "AES");
}
private static String decrypt(String encryptedData, String key) {
// 类似于encrypt()方法,只是换成Cipher.DECRYPT_MODE
// 这里省略了具体实现
}
public static void main(String[] args) {
String requestBody = "YourRequestBody"; // 需要加密的原始请求体
String encryptedRequestBody = encrypt(requestBody, KEY);
// 创建HTTP POST请求
HttpURLConnection connection = null;
try {
connection = (HttpURLConnection) new URL(URL).openConnection();
connection.setRequestMethod("POST");
connection.setRequestProperty("Content-Type", "application/json; charset=UTF-8");
connection.setRequestProperty("X-Request-ID", REQUEST_ID);
connection.setRequestProperty("Authorization", "Bearer " + encryptedRequestBody); // 假设这是一个基础的Bearer Token
// 发送请求并获取响应
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
// 如果成功,从服务器获取响应,并解密它(这一步在这里省略)
// response = decrypt(connection.getInputStream().readAllBytes(), KEY);
} else {
System.out.println("Error: " + responseCode);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if (connection != null) {
connection.disconnect();
}
}
}
}
```
阅读全文
相关推荐
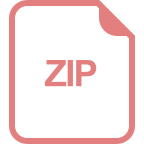
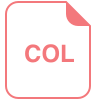
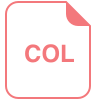
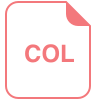
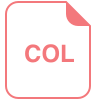
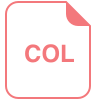
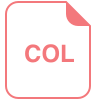
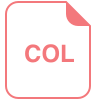
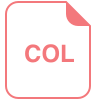
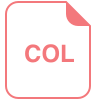
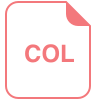
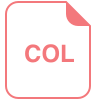
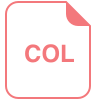
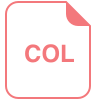
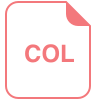
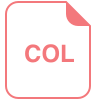
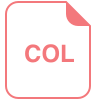
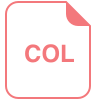