【进阶篇】数据采样与重采样:Pandas中的采样技术应用
发布时间: 2024-06-24 20:41:48 阅读量: 68 订阅数: 118 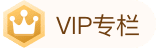
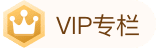
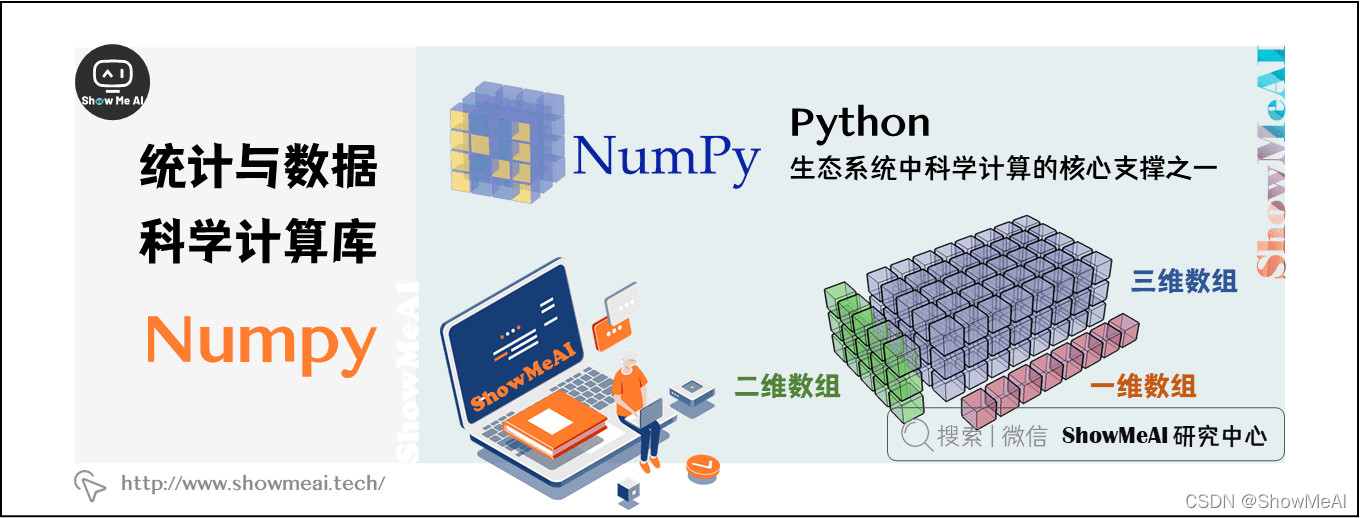
# 1. 数据采样概述
数据采样是一种从大型数据集或总体中提取代表性子集的技术。它在数据分析和建模中至关重要,因为处理整个数据集通常不可行或不切实际。数据采样允许研究人员在有限的时间和资源内获得对总体特征的见解。
数据采样方法可以分为随机采样和非随机采样。随机采样方法确保每个个体被选中的概率相等,从而产生无偏的样本。非随机采样方法根据特定标准或便利性选择个体,这可能会导致样本存在偏见。
# 2. Pandas中的数据采样技术
### 2.1 随机采样
随机采样是一种从总体中选择样本的方式,其中每个个体都有相等的机会被选中。这确保了样本代表总体,并且可以用来对总体进行推断。
#### 2.1.1 简单随机采样
简单随机采样是最基本的随机采样方法。它涉及从总体中随机选择样本,每个个体都有相等的机会被选中。这可以通过使用随机数生成器或随机抽样工具来实现。
**代码块:**
```python
import random
population = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
sample_size = 5
# 使用随机数生成器生成随机索引
random_indices = random.sample(range(len(population)), sample_size)
# 从总体中选择样本
sample = [population[i] for i in random_indices]
print(sample)
```
**逻辑分析:**
* `random.sample()` 函数从指定范围(`range(len(population))`)中生成指定数量(`sample_size`)的随机整数。
* 这些随机整数表示总体中个体的索引。
* 使用列表推导从总体中提取具有这些索引的个体,形成样本。
#### 2.1.2 分层随机采样
分层随机采样是一种随机采样方法,其中总体被分成具有相似特征的子群体(称为层)。然后,从每个层中随机选择样本。这确保了样本在每个层中具有代表性。
**代码块:**
```python
import random
population = {
"age_group": ["0-18", "19-30", "31-45", "46-60", "61+"],
"gender": ["male", "female"],
"income": ["low", "medium", "high"]
}
# 将总体划分为层
strata = list(set(population["age_group"]))
# 从每个层中随机选择样本
sample = []
for stratum in strata:
sample.extend(random.sample(population[population["age_group"] == stratum], 10))
print(sample)
```
**逻辑分析:**
* `list(set(population["age_group"]))` 获取总体中年龄组的唯一值,形成层。
* 对于每个层,使用 `random.sample()` 从该层中随机选择指定数量(10)的个体。
* 将从每个层中选择的个体添加到样本中。
#### 2.1.3 系统随机采样
系统随机采样是一种随机采样方法,其中从总体中选择一个随机起点,然后以一个固定的间隔选择样本。这确保了样本在总体中均匀分布。
**代码块:**
```python
import random
population = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
sample_size = 5
interval = 2
# 生成随机起点
random_start = random.randint(0, len(population) - sample_size)
# 以固定间隔选择样本
sample = []
for i in range(random_start, len(population), interval):
sample.append(population[i])
print(sample)
```
**逻辑分析:**
* `random.randint(0, len(population) - sample_size)` 生成一个随机起点,确保样本在总体中均匀分布。
* 使用 `range(random_start, len(population), interval)` 迭代总体,以指定的间隔选择样本。
* 将选定的个体添加到样本中。
### 2.2 非随机采样
非随机采样是一种从总体中选择样本的方式,其中某些个体比其他个体更有可能被选中。这可能导致样本不代表总体,并且不适用于对总体进行推断。
#### 2.2.1 便利采样
便利采样是一种非随机采样方法,其中样本是从容易获取的个体中选择的。这可能导致样本偏向于某些群体,并且不适用于对总体进行推断。
**代码块:**
```python
population = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
sample =
```
0
0
相关推荐
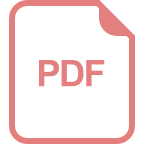
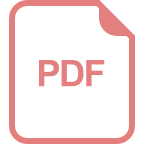





