Lucene的高级搜索与性能优化技巧
发布时间: 2024-01-13 04:26:38 阅读量: 172 订阅数: 21 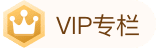
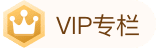
# 1. Lucene搜索引擎简介
### 1.1 Lucene的基本原理和架构
Lucene是一个开源的全文搜索引擎库,它提供了丰富的搜索功能和性能优化技巧。在深入了解Lucene的高级搜索和性能优化之前,我们首先需要了解Lucene的基本原理和架构。
Lucene的核心原理是倒排索引(Inverted Index),它通过将文档和词项的关系反转,将文档中的每个词项映射到包含该词项的文档列表上。倒排索引的结构使得搜索引擎可以快速地根据关键词进行搜索。
Lucene的架构包含以下几个关键组件:
- Analyzer(分析器):负责将文本进行分词和标准化处理,生成词项。
- IndexWriter(索引写入器):用于创建和更新索引。
- IndexReader(索引读取器):用于读取索引和执行搜索操作。
- QueryParser(查询解析器):将用户输入的查询语句解析为查询对象。
- Query(查询):表示用户的查询请求,可以是简单的词项查询,也可以是复杂的布尔查询。
### 1.2 Lucene搜索流程解析
Lucene的搜索流程可以分为以下几个步骤:
1. 创建或获取IndexReader对象。
2. 创建Query对象,表示用户的查询请求。
3. 将Query对象传递给IndexSearcher进行搜索。
4. IndexSearcher根据Query对象在倒排索引中查找匹配的文档。
5. 根据相关性进行排序,得到搜索结果。
6. 返回搜索结果给用户。
在搜索过程中,Lucene会利用倒排索引的结构和相关算法,通过严格匹配、模糊匹配、权重设置等方式来提高搜索的准确性和效率。
### 1.3 Lucene中的索引和查询
在Lucene中,索引是指将文档转换为可被搜索的数据结构。Lucene的索引是基于倒排索引的,在创建索引时,需要先对文档进行分词和标准化处理,然后将词项和文档之间的关系存储到倒排索引中。
查询是指用户提供的搜索请求。Lucene支持多种类型的查询,包括词项查询、短语查询、通配符查询、范围查询等。用户可以通过构建不同类型的查询对象,来实现精确匹配、模糊查询、多字段搜索等功能。
总结起来,Lucene搜索引擎利用倒排索引的原理和相关算法,通过索引和查询的相互配合,实现高效、准确的全文搜索功能。在接下来的章节中,我们将深入探讨Lucene的高级搜索技巧和性能优化策略。
# 2. Lucene查询语法及高级搜索技巧
### 2.1 基本查询语法和操作符
在Lucene中,查询语法是用来指定搜索条件和操作符的语言。通过灵活的查询语法,我们可以更精确地匹配和过滤搜索结果,以达到我们期望的搜索效果。下面是一些常用的查询语法和操作符:
- **Term查询**: Term查询是最基础的查询方式,它用于精确匹配一个词项,例如搜索某个特定的单词或短语。示例代码如下:
```java
String searchTerm = "lucene";
Query query = new TermQuery(new Term("content", searchTerm));
```
- **通配符查询**: 通配符查询允许使用通配符来匹配词项。通配符 `*` 表示任意字符序列,`?` 表示任意单个字符。示例代码如下:
```java
String searchTerm = "lu*ne";
Query query = new WildcardQuery(new Term("content", searchTerm));
```
- **模糊查询**: 模糊查询用于匹配与搜索项相似的词项。它可以通过设置模糊匹配的最大编辑距离来调整匹配程度。示例代码如下:
```java
String searchTerm = "lucene~";
Query query = new FuzzyQuery(new Term("content", searchTerm));
```
- **范围查询**: 范围查询用于匹配指定范围内的词项。可以使用数值、日期等类型的字段进行范围查询。示例代码如下:
```java
TermRangeQuery query = TermRangeQuery.newStringRange("date", "2019-01-01", "2020-01-01", true, true);
```
- **短语查询**: 短语查询用于匹配包含指定短语的文档。示例代码如下:
```java
String[] searchTerms = {"lucene", "search"};
Query query = new PhraseQuery.Builder().add(new Term("content", searchTerms[0])).add(new Term("content", searchTerms[1])).build();
```
- **布尔查询**: 布尔查询用于组合多个查询条件,支持与、或、非等逻辑操作符。示例代码如下:
```java
TermQuery query1 = new TermQuery(new Term("content", "lucene"));
TermQuery query2 = new TermQuery(new Term("content", "search"));
BooleanQuery.Builder builder = new BooleanQuery.Builder();
builder.add(query1, BooleanClause.Occur.MUST);
builder.add(query2, BooleanClause.Occur.MUST);
Query query = builder.build();
```
这些只是Lucene查询语法中的一小部分,通过组合和灵活运用这些查询语法和操作符,我们能够构建出更强大、更精确的查询条件来满足不同的搜索需求。
### 2.2 精确匹配和模糊查询
在实际应用中,我们常常需要进行精确匹配和模糊查询来提高搜索的准确性和灵活性。Lucene提供了多种方式来实现这些查询需求。下面我们分别介绍精确匹配和模糊查询的用法。
#### 2.2.1 精确匹配
精确匹配是指搜索结果必须完全匹配搜索项。Lucene中的TermQuery可以实现精确匹配,它会按照词项进行搜索。
示例代码如下(Java):
```java
String searchTerm = "lucene";
Query query = new TermQuery(new Term("content", searchTerm));
```
在上面的示例中,我们使用TermQuery来创建一个精确匹配查询,搜索字段为
0
0
相关推荐
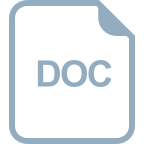
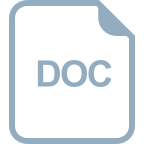
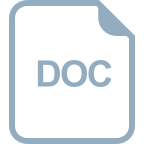
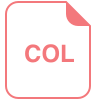

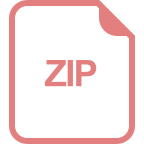
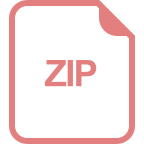
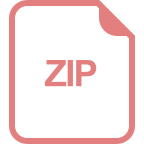
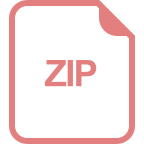